def forward(self, state): a = torch.tanh(self.l1(state.float())) a = self.bn1(a) a = torch.tanh(self.l2(a)) a = self.bn2(a) a = torch.tanh(self.l3(a)) current_power_t = self.compute_power(a.detach()).expand(-1, 2 * self.M ** 2) / np.sqrt(self.power_t) real_normal, imag_normal = self.compute_phase(a.detach()) real_normal = real_normal.expand(-1, self.N) imag_normal = imag_normal.expand(-1, self.N) division_term = torch.cat([current_power_t, real_normal, imag_normal], dim=1) return self.max_action * a / division_term逐行解释一下这段代码
时间: 2023-06-16 21:01:58 浏览: 84
这段代码是一个神经网络模型的前向传播过程,逐行解释如下:
1. `def forward(self, state):`: 定义了一个名为 forward 的函数,输入为 state(状态)。
2. `a = torch.tanh(self.l1(state.float()))`: 对输入的状态 state 进行一次全连接层操作 self.l1,并使用 tanh 函数进行激活,得到输出 a。
3. `a = self.bn1(a)`: 对输出 a 进行一次批量归一化操作 self.bn1。
4. `a = torch.tanh(self.l2(a))`: 对批量归一化后的 a 进行第二次全连接层操作 self.l2,并使用 tanh 函数进行激活,得到输出 a。
5. `a = self.bn2(a)`: 对输出 a 进行一次批量归一化操作 self.bn2。
6. `a = torch.tanh(self.l3(a))`: 对批量归一化后的 a 进行第三次全连接层操作 self.l3,并使用 tanh 函数进行激活,得到输出 a。
7. `current_power_t = self.compute_power(a.detach()).expand(-1, 2 * self.M ** 2) / np.sqrt(self.power_t)`: 调用 self.compute_power 方法计算出当前状态下的电力值 current_power_t,并将其扩展成一个大小为 (-1, 2 * self.M ** 2) 的张量,然后除以 np.sqrt(self.power_t)。
8. `real_normal, imag_normal = self.compute_phase(a.detach())`: 调用 self.compute_phase 方法计算出当前状态下的相角值 real_normal 和 imag_normal。
9. `real_normal = real_normal.expand(-1, self.N)`: 将相角值 real_normal 扩展成一个大小为 (-1, self.N) 的张量。
10. `imag_normal = imag_normal.expand(-1, self.N)`: 将相角值 imag_normal 扩展成一个大小为 (-1, self.N) 的张量。
11. `division_term = torch.cat([current_power_t, real_normal, imag_normal], dim=1)`: 将 current_power_t、real_normal 和 imag_normal 沿着列方向拼接起来,形成一个大小为 (-1, 2 * self.M ** 2 + 2 * self.N) 的张量 division_term。
12. `return self.max_action * a / division_term`: 将输出 a 乘以一个最大动作值 self.max_action,并将其除以 division_term,得到最终的输出结果。
相关推荐
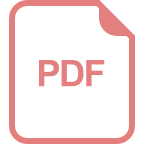
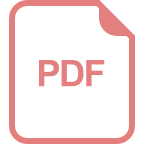















