写一个C语言程序, 给定一个有序序列,使用二分查找指定的值。 (递归/非递归两种方法)
时间: 2024-02-06 18:10:38 浏览: 38
非递归实现二分查找:
```c
#include <stdio.h>
int binary_search(int arr[], int size, int target) {
int left = 0, right = size - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target)
return mid;
else if (arr[mid] < target)
left = mid + 1;
else
right = mid - 1;
}
return -1;
}
int main() {
int arr[] = {1, 3, 4, 6, 8, 10};
int size = sizeof(arr) / sizeof(arr[0]);
int target = 6;
int index = binary_search(arr, size, target);
if (index == -1)
printf("Not found!\n");
else
printf("Found at index %d\n", index);
return 0;
}
```
递归实现二分查找:
```c
#include <stdio.h>
int binary_search_recursive(int arr[], int left, int right, int target) {
if (left > right)
return -1;
int mid = left + (right - left) / 2;
if (arr[mid] == target)
return mid;
else if (arr[mid] < target)
return binary_search_recursive(arr, mid + 1, right, target);
else
return binary_search_recursive(arr, left, mid - 1, target);
}
int main() {
int arr[] = {1, 3, 4, 6, 8, 10};
int size = sizeof(arr) / sizeof(arr[0]);
int target = 6;
int index = binary_search_recursive(arr, 0, size - 1, target);
if (index == -1)
printf("Not found!\n");
else
printf("Found at index %d\n", index);
return 0;
}
```
相关推荐
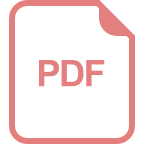
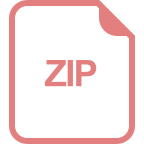
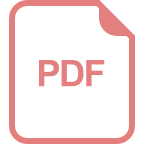














