def play(strategy0, strategy1, goal=GOAL_SCORE): """Simulate a game and return the final scores of both players, with Player 0's score first, and Player 1's score second. A strategy is a function that takes two total scores as arguments (the current player's score, and the opponent's score), and returns a number of dice that the current player will roll this turn. strategy0: The strategy function for Player 0, who plays first. strategy1: The strategy function for Player 1, who plays second. """ who = 0 # Which player is about to take a turn, 0 (first) or 1 (second) score, opponent_score = 0, 0 "*** YOUR CODE HERE ***" while score < goal and opponent_score < goal: if who == 0: score += take_turn(strategy0(score, opponent_score), opponent_score, select_dice(score, opponent_score)) if can_swap(score, opponent_score): # score = opponent_score # opponent_score = score score, opponent_score = opponent_score, score who = other(0) else: opponent_score += take_turn(strategy1(opponent_score, score), score, select_dice(opponent_score, score)) if can_swap(opponent_score, score): # opponent_score = score # score = opponent_score opponent_score, score = score, opponent_score who = other(1) return score, opponent_score换一种写法
时间: 2024-03-21 11:44:41 浏览: 24
下面是一种使用更加简洁的写法:
```
def play(strategy0, strategy1, goal=GOAL_SCORE):
scores = [0, 0]
who = 0
while max(scores) < goal:
opponent_score = scores[1 - who]
num_rolls = strategy0(scores[who], opponent_score) if who == 0 else strategy1(scores[who], opponent_score)
scores[who] += take_turn(num_rolls, opponent_score, select_dice(scores[who], opponent_score))
if can_swap(scores[who], opponent_score):
scores[who], scores[1 - who] = scores[1 - who], scores[who]
who = 1 - who
return tuple(scores)
```
这个写法将玩家的得分放在一个列表中,使用了更加简洁的语法来交替玩家的回合,并且使用了 `1 - who` 来表示另一个玩家的位置。同时,也将 `take_turn` 函数的参数与 `strategy` 函数的参数分开了,让代码更加清晰易懂。
相关问题
### Problem 6 Update your `play` function so that a commentary function is called at the end of each turn. The return value of calling a commentary function gives you the commentary function to call on the next turn. For example, `say(score0, score1)` from the parameters of the function `play` should be called at the end of the first turn. Its return value (another commentary function) should be called at the end of the second turn. Each consecutive turn, call the function that was returned by the call to the previous turn's commentary function.
Here is an updated version of the `play` function that implements the required functionality:
```python
def play(strategy0, strategy1, score0=0, score1=0, goal=GOAL_SCORE, say=say):
"""Simulate a game and return the final scores of both players, with commentary."""
player = 0
commentary = say(score0, score1)
while score0 < goal and score1 < goal:
if player == 0:
score0 += take_turn(strategy0(score0, score1), score1, goal)
else:
score1 += take_turn(strategy1(score1, score0), score0, goal)
player = other(player)
commentary = commentary(score0, score1)
return score0, score1
```
We now keep track of the `commentary` function, which is initially set to the commentary function returned by the first call to `say`. At the end of each turn, we call the `commentary` function with the new scores, and update `commentary` to the function it returns, which will be called at the end of the next turn. This continues until the game ends.
def set_data(df_0, df_1, df_9, cfg_dict): cfg_train_dict = cfg_dict['train'] df_train_1 = df_1.sample(len(df_1) - int(cfg_train_dict['simulate_pos_count']), random_state=int(cfg_train_dict['random_state'])) print('df_train_1 : ',len(df_train_1)) if cfg_train_dict['use_neg_sample'] == 'True': df_train_0 = df_0.copy() if len(df_0) >= len(df_1): df_train_0 = df_0.sample(len(df_1)) #else: # df_train_0 = df_0.append(df_9.sample(len(df_train_1) - len(df_0), # random_state=int(cfg_train_dict['random_state'])), # sort=False) else: df_train_0 = df_9.sample(round(len(df_train_1)), random_state=int(cfg_train_dict['random_state'])) df_train_0['label'] = 0 print('train set: pos_num--%i nag_num--%i' % (len(df_train_1), len(df_train_0))) df_train = df_train_1.append(df_train_0, sort=False) df_1_final_test = df_1.loc[list(set(df_1.index.tolist()).difference(set(df_train_1.index.tolist())))] #df_9_final_test = df_9.copy() 使负样本验证集等于正样本的验证集 df_9_final_test = df_9.sample(round(len(df_1_final_test)), random_state=int(cfg_train_dict['random_state'])) df_9_final_test['label'] = 0 df_ft = df_1_final_test.append(df_9_final_test, sort=False)
这段代码是一个名为 set_data 的函数,它用于设置数据集。
首先,函数从 cfg_dict 字典中获取了一个名为 cfg_train_dict 的子字典,其中包含了训练配置的相关参数。
接着,函数从 df_1 数据框中随机抽样了一部分样本作为训练集 df_train_1,抽样数量为 len(df_1) 减去 cfg_train_dict['simulate_pos_count'],random_state 参数用于设置随机种子。函数打印了 df_train_1 的长度。
然后,根据 cfg_train_dict['use_neg_sample'] 的值,函数决定如何选择负例样本。如果值为 'True',则将 df_0 数据框复制给 df_train_0,并根据 df_1 的长度进行抽样。如果 df_0 的长度大于等于 df_1 的长度,则抽样数量为 len(df_1);否则,函数还会从 df_9 数据框中抽样剩余的样本,抽样数量为 len(df_train_1) 减去 len(df_0),random_state 参数同样用于设置随机种子。
如果 cfg_train_dict['use_neg_sample'] 的值不为 'True',则直接从 df_9 数据框中抽样数量为 round(len(df_train_1)) 的样本作为负例样本,并将其赋值给 df_train_0。
接下来,函数给 df_train_0 添加了一个名为 'label' 的列,并将所有行的值都设置为 0。函数打印了 df_train_1 和 df_train_0 的长度。
然后,函数将 df_train_1 和 df_train_0 两个数据框按行合并成一个新的数据框 df_train。
接着,函数根据 df_train_1 的索引和 df_1 的索引的差异,获取了 df_1 中不在训练集中的样本,并将其赋值给 df_1_final_test。
接下来,函数从 df_9 数据框中随机抽样数量为 round(len(df_1_final_test)) 的样本作为负例测试集,并给其添加一个名为 'label' 的列,所有行的值都设置为 0。
最后,函数将 df_1_final_test 和 df_9_final_test 两个数据框按行合并成一个新的数据框 df_ft。
这段代码的作用是根据配置参数设置训练集和测试集。训练集由正例样本和负例样本组成,而测试集则包含了未在训练集中出现的正例样本和负例样本。
相关推荐
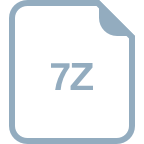
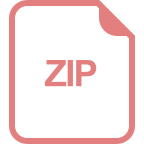
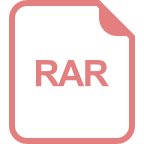













