加权无向图的最大连通分量python
时间: 2023-09-12 22:05:01 浏览: 69
### 回答1:
你好!针对你的问题,可以使用Kruskal算法来解决加权无向图的最大连通分量问题。下面是Python代码实现:
```python
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = []
def add_edge(self, u, v, w):
self.graph.append([u, v, w])
def find(self, parent, i):
if parent[i] == i:
return i
return self.find(parent, parent[i])
def union(self, parent, rank, x, y):
xroot = self.find(parent, x)
yroot = self.find(parent, y)
if rank[xroot] < rank[yroot]:
parent[xroot] = yroot
elif rank[xroot] > rank[yroot]:
parent[yroot] = xroot
else:
parent[yroot] = xroot
rank[xroot] += 1
def kruskal(self):
result = []
i = 0
e = 0
self.graph = sorted(self.graph, key=lambda item: item[2], reverse=True)
parent = []
rank = []
for node in range(self.V):
parent.append(node)
rank.append(0)
while e < self.V - 1:
u, v, w = self.graph[i]
i = i + 1
x = self.find(parent, u)
y = self.find(parent, v)
if x != y:
e = e + 1
result.append([u, v, w])
self.union(parent, rank, x, y)
return result
```
这里通过Kruskal算法实现了对加权无向图的最大连通分量的求解。在这个算法中,首先将图按边的权值从大到小排序,然后依次选择边判断是否构成环,如果不构成环,则将其加入最大连通分量中,直到找到V-1条边或者所有边都被处理完毕为止。
使用时,可以先创建一个Graph对象,然后调用add_edge方法添加图中的边,最后调用kruskal方法求解最大连通分量。
### 回答2:
加权无向图的最大连通分量问题,可以通过深度优先搜索(DFS)或广度优先搜索(BFS)来解决。
首先,我们需要定义一个图的类,表示加权无向图,并提供相应的方法来构建图和进行搜索。在构建图的过程中,我们需要存储顶点和它们之间的边的关系以及权重。
接下来,我们可以使用DFS或BFS来搜索最大连通分量。以DFS为例,首先初始化一个数组visited,用于记录每个顶点是否已经被访问过。然后,我们从图中的每个顶点开始进行搜索,每次搜索得到的连通分量都是当前最大的连通分量。具体步骤如下:
1. 初始化一个空的最大连通分量集合max_component。
2. 对于图中的每个顶点v,如果v未被访问过,执行以下步骤:
- 初始化一个空的连通分量集合component。
- 将v标记为已访问。
- 使用DFS从v开始对图进行搜索,将搜索到的所有未访问过的顶点添加到component中。
- 如果component的权重大于max_component的权重,则将component赋值给max_component。
3. 返回max_component。
下面是一个使用DFS来解决加权无向图最大连通分量问题的Python代码段:
```
class Graph:
def __init__(self, vertices):
self.V = vertices
self.adj = [[] for _ in range(vertices)]
def addEdge(self, u, v, w):
self.adj[u].append((v, w))
self.adj[v].append((u, w))
def DFS(self, v, visited, component):
visited[v] = True
component.append(v)
for neighbor, _ in self.adj[v]:
if not visited[neighbor]:
self.DFS(neighbor, visited, component)
def findMaxComponent(self):
visited = [False] * self.V
max_component = []
for v in range(self.V):
if not visited[v]:
component = []
self.DFS(v, visited, component)
if len(component) > len(max_component):
max_component = component
return max_component
```
以上代码中,我们定义了一个Graph类,包含了构建图和执行DFS搜索的方法。使用addEdge方法构建图,使用findMaxComponent方法来找到最大连通分量。
最后,我们可以按照以下方式使用这个图类来解决问题:
```
g = Graph(4)
g.addEdge(0, 1, 3)
g.addEdge(1, 2, 5)
g.addEdge(2, 3, 2)
max_component = g.findMaxComponent()
print("最大连通分量:", max_component)
```
上述代码中,我们创建了一个包含4个顶点的加权无向图,并添加了3条边。然后,我们调用findMaxComponent方法找到图的最大连通分量,并将结果打印输出。
希望上述解答对你有帮助!
### 回答3:
加权无向图的最大连通分量,是指在一个加权无向图中,找到一个子图,使得这个子图中的所有节点之间都有通路,并且这个子图的总权重最大。
在Python中,可以使用深度优先搜索(DFS)或广度优先搜索(BFS)的方式来解决这个问题。以下是使用DFS的实现方法:
1. 首先,定义一个函数来执行DFS:
```
def DFS(graph, visited, start, max_weight, current_weight, component):
visited[start] = True
current_weight += graph[start][start] # 累加当前节点的权重
if current_weight > max_weight:
max_weight = current_weight # 更新最大权重
component.append(start) # 将当前节点添加到连通分量中
for neighbor in graph[start]:
if not visited[neighbor]:
max_weight, current_weight, component = DFS(graph, visited, neighbor, max_weight, current_weight, component)
return max_weight, current_weight, component
```
2. 然后,定义一个函数来查找最大连通分量:
```
def find_max_connected_component(graph):
max_weight = 0 # 最大权重
max_component = [] # 最大连通分量
visited = [False] * len(graph) # 记录节点是否已访问
for node in range(len(graph)):
if not visited[node]:
current_weight = 0 # 当前连通分量的权重
component = [] # 当前连通分量
max_weight, current_weight, component = DFS(graph, visited, node, max_weight, current_weight, component)
if current_weight > max_weight:
max_weight = current_weight
max_component = component
return max_component
```
3. 最后,使用上述函数来查找最大连通分量:
```
graph = [
[0, 2, 3, 0],
[2, 0, 0, 4],
[3, 0, 0, 5],
[0, 4, 5, 0]
]
max_connected_component = find_max_connected_component(graph)
print(max_connected_component)
```
上述代码中,graph表示一个加权无向图的邻接矩阵,其中0表示两个节点之间没有边,非零数字表示边的权重。函数find_max_connected_component返回最大连通分量的节点列表。在上述示例中,最大连通分量节点列表为[0, 1, 3],对应的节点权重总和为6。
相关推荐
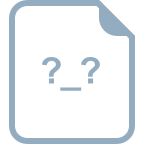
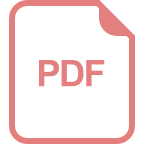
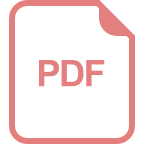
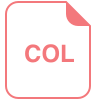













