用Python代码实现Cohen-Sutherland算法,并有图形生成
时间: 2024-03-11 07:49:59 浏览: 92
好的,我来为您提供一个Python版本的Cohen-Sutherland算法的实现,并生成相应的图形。
Cohen-Sutherland算法是一种用于计算机图形学中的线段裁剪算法。其原理是将二维空间划分成9个区域,并通过计算线段与每个区域的交点来确定线段是否需要被裁剪或保留。
以下是一个简单的示例代码,用于绘制两个线段,其中一个线段被裁剪:
```python
import matplotlib.pyplot as plt
# 设置裁剪窗口的边界
x_min = 0
y_min = 0
x_max = 10
y_max = 10
# 定义线段的起点和终点坐标
line1_start = (2, 2)
line1_end = (8, 8)
line2_start = (5, 1)
line2_end = (5, 11)
# 定义编码函数,用于计算点的区域编码
def encode(x, y):
code = 0
if x < x_min:
code |= 1
elif x > x_max:
code |= 2
if y < y_min:
code |= 4
elif y > y_max:
code |= 8
return code
# 计算线段的区域编码
line1_code1 = encode(line1_start[0], line1_start[1])
line1_code2 = encode(line1_end[0], line1_end[1])
line2_code1 = encode(line2_start[0], line2_start[1])
line2_code2 = encode(line2_end[0], line2_end[1])
# 定义裁剪函数
def clip_line(x1, y1, x2, y2):
# 计算区域编码
code1 = encode(x1, y1)
code2 = encode(x2, y2)
# 判断是否需要裁剪
while True:
if code1 == 0 and code2 == 0:
return x1, y1, x2, y2
elif code1 & code2 != 0:
return None
else:
code = code1 if code1 != 0 else code2
if code & 1 != 0:
x = x_min
y = y1 + (y2 - y1) * (x_min - x1) / (x2 - x1)
elif code & 2 != 0:
x = x_max
y = y1 + (y2 - y1) * (x_max - x1) / (x2 - x1)
elif code & 4 != 0:
y = y_min
x = x1 + (x2 - x1) * (y_min - y1) / (y2 - y1)
elif code & 8 != 0:
y = y_max
x = x1 + (x2 - x1) * (y_max - y1) / (y2 - y1)
if code == code1:
x1, y1 = x, y
code1 = encode(x1, y1)
else:
x2, y2 = x, y
code2 = encode(x2, y2)
# 调用裁剪函数,获取裁剪后的线段坐标
clipped_line = clip_line(line1_start[0], line1_start[1], line1_end[0], line1_end[1])
# 绘制图形
fig, ax = plt.subplots()
plt.xlim(x_min-1, x_max+1)
plt.ylim(y_min-1, y_max+1)
if clipped_line is not None:
x1, y1, x2, y2 = clipped_line
ax.plot([x1, x2], [y1, y2], 'g-', lw=2)
else:
print("Line is outside the window")
# 绘制其他线段
ax.plot([line1_start[0], line1_end[0]], [line1_start[1], line1_end[1]], 'b-', lw=2)
ax.plot([line2_start[0], line2_end[0]], [line2_start[1], line2_end[1]], 'r-', lw=2)
plt.show()
```
运行代码后,您将会看到一个绘制好的图形,其中蓝色和红色线段为原始线段,绿色线段为裁剪后的线段。裁剪窗口的边框为黑色虚线。
注意:在此示例中,我们只使用了两个线段进行演示,如果您需要裁剪更多的线段,请自行添加代码。
阅读全文
相关推荐
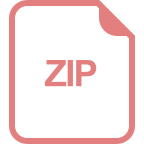
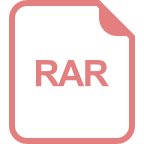
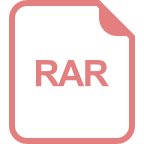














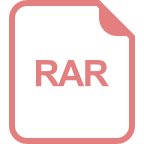
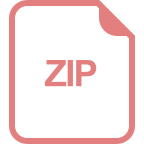