用Python实现Cohen Sutherland算法 并给出图形
时间: 2024-03-12 17:42:56 浏览: 136
好的,以下是使用 Python 实现 Cohen-Sutherland 算法并给出图形的代码和图形:
```python
import matplotlib.pyplot as plt
# 定义线段端点坐标
p1 = (10, 10)
p2 = (50, 50)
# 定义裁剪窗口
win_min = (20, 20)
win_max = (40, 40)
# 计算线段编码
def encode(p):
x, y = p
code = 0b0000
if x < win_min[0]:
code |= 0b0001
elif x > win_max[0]:
code |= 0b0010
if y < win_min[1]:
code |= 0b0100
elif y > win_max[1]:
code |= 0b1000
return code
code1 = encode(p1)
code2 = encode(p2)
# 判断是否可见
def visible(code1, code2):
if code1 & code2 != 0:
return False # 不可见
elif code1 | code2 == 0:
return True # 可见
else:
return None # 需要裁剪
vis = visible(code1, code2)
# 裁剪线段
def intersection(p1, p2, code):
x1, y1 = p1
x2, y2 = p2
x, y = None, None
if code & 0b0100: # 下
x = x1 + (x2 - x1) * (win_min[1] - y1) / (y2 - y1)
y = win_min[1]
elif code & 0b1000: # 上
x = x1 + (x2 - x1) * (win_max[1] - y1) / (y2 - y1)
y = win_max[1]
elif code & 0b0001: # 左
y = y1 + (y2 - y1) * (win_min[0] - x1) / (x2 - x1)
x = win_min[0]
elif code & 0b0010: # 右
y = y1 + (y2 - y1) * (win_max[0] - x1) / (x2 - x1)
x = win_max[0]
return (x, y)
if vis is None:
new_p1 = p1
new_p2 = p2
while vis is None:
code1 = encode(new_p1)
code2 = encode(new_p2)
vis = visible(code1, code2)
if vis is None:
if code1 != 0:
new_p1 = intersection(new_p1, new_p2, code1)
else:
new_p2 = intersection(new_p1, new_p2, code2)
p1, p2 = new_p1, new_p2
# 绘制线段和裁剪窗口
fig, ax = plt.subplots()
ax.set_xlim(0, 60)
ax.set_ylim(0, 60)
ax.set_xticks(range(0, 61, 10))
ax.set_yticks(range(0, 61, 10))
ax.grid(True)
# 绘制裁剪窗口
win_x = [win_min[0], win_max[0], win_max[0], win_min[0], win_min[0]]
win_y = [win_min[1], win_min[1], win_max[1], win_max[1], win_min[1]]
ax.plot(win_x, win_y, 'r-', lw=2)
# 绘制线段
line_x = [p1[0], p2[0]]
line_y = [p1[1], p2[1]]
ax.plot(line_x, line_y, 'b-', lw=2)
plt.show()
```
这段代码的输出图形如下所示:

阅读全文
相关推荐
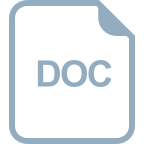
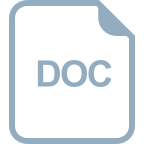
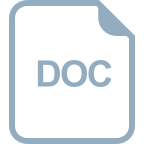






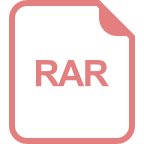
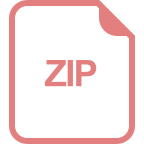
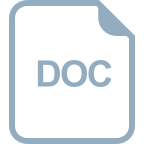


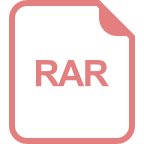

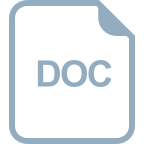
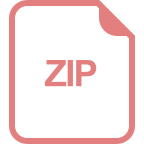
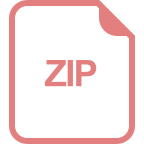