用tkinter作贪吃蛇
时间: 2024-06-20 10:02:33 浏览: 66
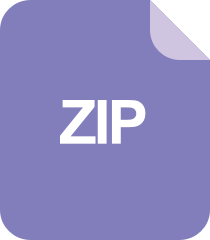
Python+tkinter制作的贪吃蛇小游戏
在Python中,使用Tkinter库创建一个简单的贪吃蛇游戏是一个很好的实践项目,它可以帮助你熟悉事件驱动编程和窗口管理器的基本概念。以下是使用Tkinter制作贪吃蛇游戏的基本步骤:
1. **导入模块**:
```python
import tkinter as tk
from tkinter import messagebox
import random
```
2. **创建主窗口**:
```python
window = tk.Tk()
window.title("贪吃蛇")
window.geometry("400x400")
```
3. **定义蛇、食物和得分**:
```python
snake_body = []
food = None
score = 0
```
4. **画布和游戏元素**:
- 创建一个Canvas对象并在窗口上绘制蛇、食物和 Score Label。
- 使用`create_rectangle`或`create_oval`方法画出形状。
5. **事件处理**:
- `snake_move` 函数:定义蛇移动的逻辑,根据方向改变蛇的位置。
- `check_collision` 函数:检测蛇是否撞到墙壁或自身。
- `eat_food` 函数:当蛇吃到食物时,增长蛇身并生成新的食物位置。
6. **键盘监听**:
```python
def key_listener(event):
global direction
if event.keycode == 37: # left
direction = "left"
elif event.keycode == 38: # up
direction = "up"
elif event.keycode == 39: # right
direction = "right"
elif event.keycode == 40: # down
direction = "down"
```
7. **游戏循环**:
```python
while True:
window.update()
for event in window.event_sequence:
key_listener(event)
snake_move()
check_collision()
eat_food()
if game_over():
break
```
8. **游戏结束**:
```python
def game_over():
if len(snake_body) >= 15 or snake_body == food:
messagebox.showinfo("Game Over", "Your score is: " + str(score))
return True
```
9. **启动游戏循环**:
```python
window.bind("<Key>", key_listener)
```
阅读全文
相关推荐
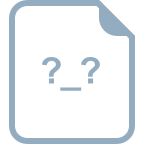
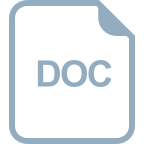




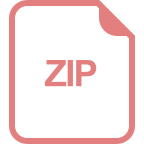
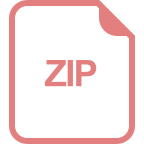
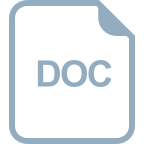


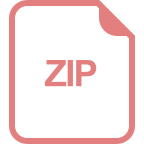
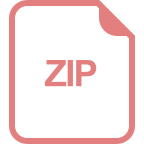
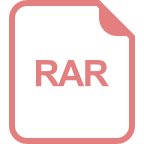
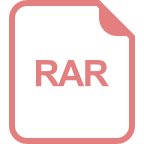
