C++ 求取6*6矩阵协方差及特征值
时间: 2023-07-24 16:31:34 浏览: 102
以下是使用 C++ 求取 6*6 矩阵协方差和特征值的示例代码:
```c++
#include <iostream>
#include <cmath>
#include <iomanip>
using namespace std;
// 求取矩阵均值
void getMean(double matrix[6][6], double mean[6]) {
for (int j = 0; j < 6; j++) {
double sum = 0;
for (int i = 0; i < 6; i++) {
sum += matrix[i][j];
}
mean[j] = sum / 6.0;
}
}
// 求取协方差矩阵
void getCovariance(double matrix[6][6], double covariance[6][6], double mean[6]) {
for (int i = 0; i < 6; i++) {
for (int j = 0; j < 6; j++) {
double sum = 0;
for (int k = 0; k < 6; k++) {
sum += (matrix[k][i] - mean[i]) * (matrix[k][j] - mean[j]);
}
covariance[i][j] = sum / 5.0;
}
}
}
// 打印矩阵
void printMatrix(double matrix[6][6]) {
for (int i = 0; i < 6; i++) {
for (int j = 0; j < 6; j++) {
cout << setw(10) << matrix[i][j];
}
cout << endl;
}
}
// 求取特征值和特征向量
void getEigenValue(double matrix[6][6], double eigenvalue[6], double eigenvector[6][6]) {
double eps = 1e-10;
double err = 1.0;
int max_iter = 200;
int n = 6;
// 初始化特征向量
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
eigenvector[i][j] = (i == j) ? 1 : 0;
}
}
// 迭代求解特征值和特征向量
int iter = 0;
while (err > eps && iter < max_iter) {
double sum = 0;
for (int i = 0; i < n; i++) {
for (int j = i + 1; j < n; j++) {
sum += pow(matrix[i][j], 2);
}
}
err = sqrt(sum);
for (int p = 0; p < n; p++) {
for (int q = p + 1; q < n; q++) {
double app = matrix[p][p];
double apq = matrix[p][q];
double aqq = matrix[q][q];
double phi = 0.5 * atan2(2 * apq, aqq - app);
double c = cos(phi);
double s = sin(phi);
matrix[p][p] = c * c * app + 2 * c * s * apq + s * s * aqq;
matrix[q][q] = s * s * app - 2 * c * s * apq + c * c * aqq;
matrix[p][q] = 0;
matrix[q][p] = 0;
for (int k = 0; k < n; k++) {
if (k != p && k != q) {
double akp = matrix[k][p];
double akq = matrix[k][q];
matrix[k][p] = c * akp + s * akq;
matrix[p][k] = matrix[k][p];
matrix[k][q] = -s * akp + c * akq;
matrix[q][k] = matrix[k][q];
}
}
for (int k = 0; k < n; k++) {
double epi = eigenvector[k][p];
double eqi = eigenvector[k][q];
eigenvector[k][p] = c * epi + s * eqi;
eigenvector[k][q] = -s * epi + c * eqi;
}
}
}
iter++;
}
for (int i = 0; i < n; i++) {
eigenvalue[i] = matrix[i][i];
}
}
int main() {
double matrix[6][6] = {
{ 1, 2, 3, 4, 5, 6 },
{ 2, 3, 4, 5, 6, 7 },
{ 3, 4, 5, 6, 7, 8 },
{ 4, 5, 6, 7, 8, 9 },
{ 5, 6, 7, 8, 9, 10 },
{ 6, 7, 8, 9, 10, 11 }
};
double mean[6];
double covariance[6][6];
double eigenvalue[6];
double eigenvector[6][6];
// 求取矩阵均值
getMean(matrix, mean);
// 求取协方差矩阵
getCovariance(matrix, covariance, mean);
// 打印协方差矩阵
cout << "协方差矩阵:" << endl;
printMatrix(covariance);
// 求取特征值和特征向量
getEigenValue(covariance, eigenvalue, eigenvector);
// 打印特征值
cout << "特征值:" << endl;
for (int i = 0; i < 6; i++) {
cout << eigenvalue[i] << endl;
}
// 打印特征向量
cout << "特征向量:" << endl;
printMatrix(eigenvector);
return 0;
}
```
该示例代码中,首先求取矩阵均值,然后求取协方差矩阵,接着使用迭代法求取特征值和特征向量。最后打印协方差矩阵、特征值和特征向量。
阅读全文
相关推荐







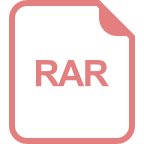








