地铁跑酷代码python
时间: 2023-10-28 21:00:01 浏览: 54
当然,这是一个简单的地铁跑酷游戏的 Python 代码示例:
```python
import pygame
import random
# 初始化
pygame.init()
# 窗口大小
screen_width = 800
screen_height = 400
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("Subway Surfers")
# 背景图片
background_image = pygame.image.load("background.png")
# 玩家图片
player_image = pygame.image.load("player.png")
player_width = 50
player_height = 100
player_x = 100
player_y = screen_height - player_height
# 障碍物图片
obstacle_image = pygame.image.load("obstacle.png")
obstacle_width = 50
obstacle_height = 50
obstacle_x = screen_width
obstacle_y = screen_height - obstacle_height
# 分数设置
score = 0
font = pygame.font.Font('freesansbold.ttf', 32)
text_x = 10
text_y = 10
# 游戏结束标志
game_over = False
clock = pygame.time.Clock()
def show_score(x, y):
score_text = font.render("Score: " + str(score), True, (255, 255, 255))
screen.blit(score_text, (x, y))
def player(x, y):
screen.blit(player_image, (x, y))
def obstacle(x, y):
screen.blit(obstacle_image, (x, y))
def check_collision(player_x, player_y, obstacle_x, obstacle_y):
if player_x + player_width > obstacle_x and player_x < obstacle_x + obstacle_width:
if player_y + player_height > obstacle_y and player_y < obstacle_y + obstacle_height:
return True
return False
# 游戏循环
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
player_y -= 50
screen.blit(background_image, (0, 0))
obstacle_x -= 5
if obstacle_x < -obstacle_width:
obstacle_x = screen_width
obstacle_y = random.randint(0, screen_height - obstacle_height)
score += 1
if check_collision(player_x, player_y, obstacle_x, obstacle_y):
game_over = True
player(player_x, player_y)
obstacle(obstacle_x, obstacle_y)
show_score(text_x, text_y)
pygame.display.update()
clock.tick(60)
# 游戏结束
pygame.quit()
```
请确保你有以下文件:
- `background.png`:背景图片
- `player.png`:玩家图片
- `obstacle.png`:障碍物图片
你可以根据自己的需求修改和完善代码。希望对你有所帮助!
相关推荐
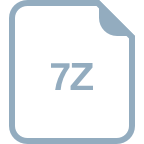
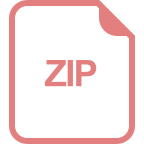














