请用c语言编写一个可以实时显示当前时间到开发板上的程序
时间: 2024-10-09 11:01:57 浏览: 30
在C语言中,实时显示当前时间并将其发送到开发板上通常涉及到硬件交互,比如通过串口通信。这里我将提供一个基本示例,假设我们正在使用Linux环境,并利用`stdio.h`, `time.h`, 和 `fcntl.h` 等头文件。如果要在嵌入式系统或特定的开发板上运行,你可能需要相应驱动支持。
```c
#include <stdio.h>
#include <time.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <linux/delay.h> // 对于延时处理
#define SERIAL_PORT "/dev/ttyS0" // 替换为你的串口设备路径
#define BAUD_RATE 9600
#define CMD "\r\nCurrent Time: %d-%02d-%02d %02d:%02d:%02d\r\n"
int main() {
int fd = open(SERIAL_PORT, O_RDWR | O_NOCTTY | O_NDELAY); // 打开串口
if (fd == -1) {
perror("Failed to open serial port");
return 1;
}
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600); // 设置波特率
cfsetospeed(&options, B9600);
options.c_cflag &= ~PARENB; // 取消奇偶校验
options.c_cflag &= ~CSTOPB; // 只用1位停止位
options.c_cflag &= ~CSIZE; // 8位数据
options.c_cflag |= CS8;
options.c_iflag &= ~(IXON | IXOFF | IXANY); // 关闭软件流控
options.c_oflag &= ~OPOST; // 没有输出流控制
options.c_lflag &= ~(ICANON | ECHO | ISIG); // 非终端模式,禁用回显
tcflush(fd, TCIOFLUSH); // 清空缓冲区
ioctl(fd, TIOCBASESET, NULL); // 设置基础设备操作
while (1) {
time_t raw_time;
struct tm *timeinfo;
time(&raw_time);
timeinfo = localtime(&raw_time);
char currentTimeStr[50];
strftime(currentTimeStr, sizeof(currentTimeStr), CMD, timeinfo);
write(fd, currentTimeStr, strlen(currentTimeStr)); // 写入字符串
usleep(1000000 / BAUD_RATE); // 暂停一段时间让信息充分发送,这里的时间单位是微秒
}
close(fd);
return 0;
}
```
阅读全文
相关推荐
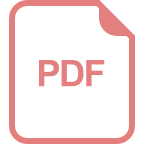
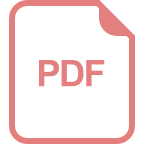
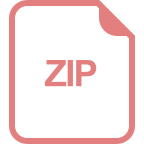
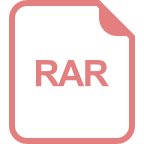
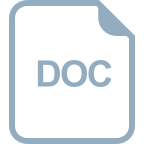


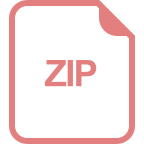
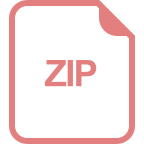
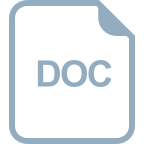
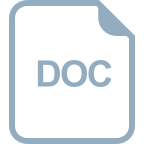
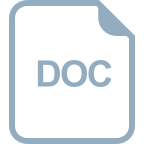
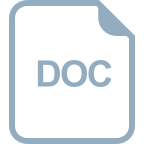
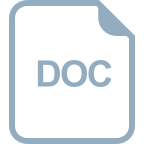
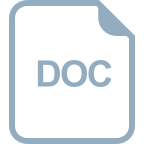



