使用c代码,easyx库实现能够人机对决的五子棋。
时间: 2023-05-11 21:02:39 浏览: 121
非常感谢您的提问!以下是使用C代码和EasyX库实现人机对决的五子棋的示例代码:
```c
#include <graphics.h>
#include <conio.h>
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define BOARD_SIZE 15
#define CELL_SIZE 40
#define BOARD_LEFT 50
#define BOARD_TOP 50
#define BOARD_RIGHT (BOARD_LEFT + BOARD_SIZE * CELL_SIZE)
#define BOARD_BOTTOM (BOARD_TOP + BOARD_SIZE * CELL_SIZE)
#define PLAYER 1
#define COMPUTER 2
int board[BOARD_SIZE][BOARD_SIZE] = {0};
int playerColor = RED;
int computerColor = YELLOW;
void drawBoard()
{
setbkcolor(WHITE);
cleardevice();
setlinestyle(PS_SOLID, 2);
setlinecolor(BLACK);
for (int i = 0; i <= BOARD_SIZE; i++)
{
line(BOARD_LEFT, BOARD_TOP + i * CELL_SIZE, BOARD_RIGHT, BOARD_TOP + i * CELL_SIZE);
line(BOARD_LEFT + i * CELL_SIZE, BOARD_TOP, BOARD_LEFT + i * CELL_SIZE, BOARD_BOTTOM);
}
setfillcolor(BLACK);
solidcircle(BOARD_LEFT + 3 * CELL_SIZE, BOARD_TOP + 3 * CELL_SIZE, 5);
solidcircle(BOARD_LEFT + 11 * CELL_SIZE, BOARD_TOP + 3 * CELL_SIZE, 5);
solidcircle(BOARD_LEFT + 7 * CELL_SIZE, BOARD_TOP + 7 * CELL_SIZE, 5);
solidcircle(BOARD_LEFT + 3 * CELL_SIZE, BOARD_TOP + 11 * CELL_SIZE, 5);
solidcircle(BOARD_LEFT + 11 * CELL_SIZE, BOARD_TOP + 11 * CELL_SIZE, 5);
}
void putChess(int x, int y, int color)
{
setfillcolor(color);
solidcircle(BOARD_LEFT + x * CELL_SIZE, BOARD_TOP + y * CELL_SIZE, CELL_SIZE / 2 - 2);
}
int checkWin(int x, int y, int color)
{
int count = 1;
for (int i = x - 1; i >= 0 && board[i][y] == color; i--)
count++;
for (int i = x + 1; i < BOARD_SIZE && board[i][y] == color; i++)
count++;
if (count >= 5)
return color;
count = 1;
for (int i = y - 1; i >= 0 && board[x][i] == color; i--)
count++;
for (int i = y + 1; i < BOARD_SIZE && board[x][i] == color; i++)
count++;
if (count >= 5)
return color;
count = 1;
for (int i = x - 1, j = y - 1; i >= 0 && j >= 0 && board[i][j] == color; i--, j--)
count++;
for (int i = x + 1, j = y + 1; i < BOARD_SIZE && j < BOARD_SIZE && board[i][j] == color; i++, j++)
count++;
if (count >= 5)
return color;
count = 1;
for (int i = x - 1, j = y + 1; i >= 0 && j < BOARD_SIZE && board[i][j] == color; i--, j++)
count++;
for (int i = x + 1, j = y - 1; i < BOARD_SIZE && j >= 0 && board[i][j] == color; i++, j--)
count++;
if (count >= 5)
return color;
return 0;
}
int evaluate(int color)
{
int score = 0;
for (int i = 0; i < BOARD_SIZE; i++)
{
for (int j = 0; j < BOARD_SIZE; j++)
{
if (board[i][j] == color)
{
int count = 1;
for (int k = i - 1; k >= 0 && board[k][j] == color; k--)
count++;
for (int k = i + 1; k < BOARD_SIZE && board[k][j] == color; k++)
count++;
if (count >= 5)
return 1000000;
count = 1;
for (int k = j - 1; k >= 0 && board[i][k] == color; k--)
count++;
for (int k = j + 1; k < BOARD_SIZE && board[i][k] == color; k++)
count++;
if (count >= 5)
return 1000000;
count = 1;
for (int k = i - 1, l = j - 1; k >= 0 && l >= 0 && board[k][l] == color; k--, l--)
count++;
for (int k = i + 1, l = j + 1; k < BOARD_SIZE && l < BOARD_SIZE && board[k][l] == color; k++, l++)
count++;
if (count >= 5)
return 1000000;
count = 1;
for (int k = i - 1, l = j + 1; k >= 0 && l < BOARD_SIZE && board[k][l] == color; k--, l++)
count++;
for (int k = i + 1, l = j - 1; k < BOARD_SIZE && l >= 0 && board[k][l] == color; k++, l--)
count++;
if (count >= 5)
return 1000000;
score += count * count;
}
}
}
return score;
}
int computerMove()
{
int maxScore = -1;
int bestX = -1, bestY = -1;
for (int i = 0; i < BOARD_SIZE; i++)
{
for (int j = 0; j < BOARD_SIZE; j++)
{
if (board[i][j] == 0)
{
board[i][j] = computerColor;
int score = evaluate(computerColor) + evaluate(playerColor);
board[i][j] = 0;
if (score > maxScore)
{
maxScore = score;
bestX = i;
bestY = j;
}
}
}
}
return bestX * BOARD_SIZE + bestY;
}
int main()
{
initgraph(BOARD_RIGHT + 50, BOARD_BOTTOM + 50);
drawBoard();
int turn = PLAYER;
while (1)
{
if (turn == PLAYER)
{
int x, y;
while (1)
{
if (_kbhit())
{
char ch = _getch();
if (ch == 27) // ESC
exit(0);
else if (ch == ' ') // SPACE
{
x = (getmousex() - BOARD_LEFT) / CELL_SIZE;
y = (getmousey() - BOARD_TOP) / CELL_SIZE;
if (x >= 0 && x < BOARD_SIZE && y >= 0 && y < BOARD_SIZE && board[x][y] == 0)
break;
}
}
}
putChess(x, y, playerColor);
board[x][y] = PLAYER;
int winner = checkWin(x, y, PLAYER);
if (winner)
{
if (winner == PLAYER)
outtextxy(BOARD_RIGHT + 10, BOARD_TOP + 100, "You win!");
else
outtextxy(BOARD_RIGHT + 10, BOARD_TOP + 100, "Computer wins!");
break;
}
turn = COMPUTER;
}
else
{
int move = computerMove();
int x = move / BOARD_SIZE;
int y = move % BOARD_SIZE;
putChess(x, y, computerColor);
board[x][y] = COMPUTER;
int winner = checkWin(x, y, COMPUTER);
if (winner)
{
if (winner == PLAYER)
outtextxy(BOARD_RIGHT + 10, BOARD_TOP + 100, "You win!");
else
outtextxy(BOARD_RIGHT + 10, BOARD_TOP + 100, "Computer wins!");
break;
}
turn = PLAYER;
}
}
while (!_kbhit())
Sleep(100);
closegraph();
return 0;
}
```
希望这个示例代码能够帮助您实现人机对决的五子棋!
阅读全文
相关推荐
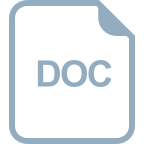
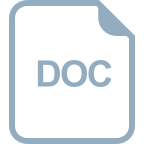
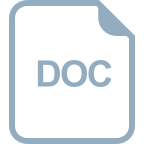
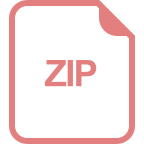
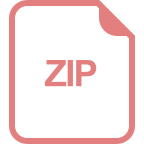
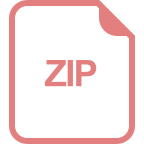
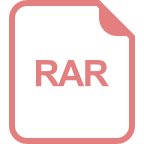
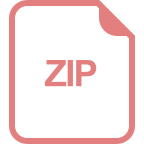
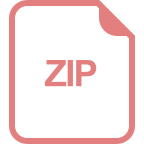
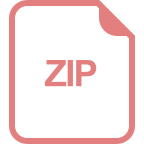



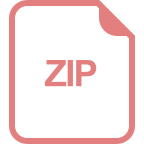
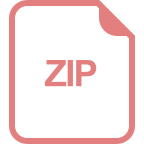
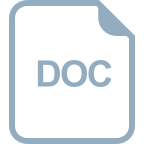

