switch(bits) { case 5: options.c_cflag &= ~CSIZE; options.c_cflag |= CS5; break; case 6: options.c_cflag &= ~CSIZE; options.c_cflag |= CS6; break; case 7: options.c_cflag &= ~CSIZE; options.c_cflag |= CS7; break; case 8: options.c_cflag &= ~CSIZE; options.c_cflag |= CS8; break; default: fprintf(stderr,"Unkown bits!\n"); return -1; }
时间: 2024-02-10 22:07:40 浏览: 25
这段代码是一个 switch 语句,根据变量 bits 的不同取值执行不同的操作。
当 bits 的值为 5 时,会将 options.c_cflag 中的 CSIZE 标志位清零,并将 CS5 标志位置为 1;当 bits 的值为 6 时,也会将 CSIZE 标志位清零,并将 CS6 标志位置为 1;当 bits 的值为 7 时,同样会将 CSIZE 标志位清零,并将 CS7 标志位置为 1;当 bits 的值为 8 时,同样会将 CSIZE 标志位清零,并将 CS8 标志位置为 1。如果 bits 的值不在 5、6、7、8 之间,则会输出错误信息并返回 -1。
这段代码的作用是根据数据位的不同设置串口通信参数。其中,CSIZE 是数据位掩码,CS5、CS6、CS7 和 CS8 分别表示数据位为 5、6、7、8。
相关问题
options.c_cflag &= ~PARENB; options.c_iflag &= ~INPCK;
这两行代码是用来设置串口的奇偶校验位(Parity Bit)的,其中 `options` 是一个 `termios` 结构体,`c_cflag` 和 `c_iflag` 都是其中的成员,分别表示终端的控制信息和输入控制信息。`PARENB` 和 `INPCK` 都是常量,分别表示启用奇偶校验和输入奇偶校验。
第一行代码 `options.c_cflag &= ~PARENB` 的作用是将 `options` 中的 `c_cflag` 成员的值与 `PARENB` 的反码进行按位与操作,来将奇偶校验位设置为关闭状态,即不使用奇偶校验。第二行代码 `options.c_iflag &= ~INPCK` 的作用是将 `options` 中的 `c_iflag` 成员的值与 `INPCK` 的反码进行按位与操作,来将输入奇偶校验设置为关闭状态。
奇偶校验是一种用于检测和纠正串口传输中数据位错误的方法。在传输数据时,发送方会在数据位后面添加一个奇偶校验位,用于表示数据位中1的个数是奇数或偶数。接收方在接收到数据后,会重新计算数据位中1的个数,并与接收到的奇偶校验位进行比较,如果不一致,则说明数据位出现了错误。但是,启用奇偶校验会增加数据传输的时间和复杂度,因此在不需要高可靠性的情况下,一般会关闭奇偶校验。
struct termios options; tcgetattr(fd, &options); options.c_cflag |= ( CLOCAL | CREAD ); options.c_cflag &= ~CSIZE; options.c_cflag &= ~CRTSCTS; options.c_cflag |= CS8; options.c_cflag &= ~CSTOPB; options.c_iflag |= IGNPAR; options.c_iflag &= ~(ICRNL | IXON); options.c_oflag = 0; options.c_lflag = 0; cfsetispeed(&options, B115200); cfsetospeed(&options, B115200); tcsetattr(fd,TCSANOW,&options); sleep(1); tcflush(fd, TCIOFLUSH);
这段代码是用于设置串口的参数。让我逐行解释一下每个步骤的作用:
1. `struct termios options;`:定义一个 `termios` 结构体,用于存储串口参数。
2. `tcgetattr(fd, &options);`:获取当前串口的参数,并将其保存在 `options` 结构体中。
3. `options.c_cflag |= ( CLOCAL | CREAD );`:设置控制模式标志,使得串口能够工作。
4. `options.c_cflag &= ~CSIZE;`:清除数据位设置的标志位。
5. `options.c_cflag &= ~CRTSCTS;`:清除硬件流控制标志位。
6. `options.c_cflag |= CS8;`:设置数据位为8位。
7. `options.c_cflag &= ~CSTOPB;`:设置停止位为1位。
8. `options.c_iflag |= IGNPAR;`:忽略奇偶校验错误的数据。
9. `options.c_iflag &= ~(ICRNL | IXON);`:禁用输入数据的回车换行转换和软件流控制。
10. `options.c_oflag = 0;`:禁用输出数据的处理。
11. `options.c_lflag = 0;`:设置本地模式标志位为0,禁用终端模式的特殊字符处理。
12. `cfsetispeed(&options, B115200);`:设置输入波特率为115200。
13. `cfsetospeed(&options, B115200);`:设置输出波特率为115200。
14. `tcsetattr(fd,TCSANOW,&options);`:将修改后的串口参数设置到串口中。
15. `sleep(1);`:延时1秒,确保参数生效。
16. `tcflush(fd, TCIOFLUSH);`:清空输入输出缓冲区,丢弃所有未读写的数据。
这段代码的作用是设置串口的工作参数,并通过 `tcsetattr` 函数将参数应用到串口上。最后,清空缓冲区以确保数据的一致性。
相关推荐
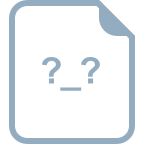
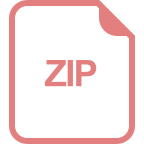












