1、父进程使用系统调用pipe()建立一个管道,然后使用系统调用fork()创建两个子进程:子进程1和子进程2 子进程1每隔1秒通过管道向子进程2发送数据:I send message x times.(x初值为1,以后发送一次后做加一操作)子进程2从管道读出信息,并显示在屏幕上 父进程用系统调用signal()来捕捉来自键盘的中断信号SIGINT(即按Ctrl+C键,);当捕捉到中断信号后,父进程用系统调用kill()向两个子进程发出信号,子进程捕捉到信号后分别输出如下信息后终止: Child Process 1 is killed by Parent! Child Process 2 is killed by Parent! 父进程等待两个子进程终止后,释放管道并输出如下的信息后终止 Parent Process is Killed! 2、利用fork结合exec启动某一进程,如系统命令,或自己写的一个测试程序。
时间: 2023-05-11 18:03:57 浏览: 94
对于第一个问题,以下是可能的解决方案:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <signal.h>
#define MSG_SIZE 20
int main() {
int fd[2];
pid_t pid1, pid2;
int x = 1;
char msg[MSG_SIZE];
if (pipe(fd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
pid1 = fork();
if (pid1 == -1) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid1 == 0) { // child process 1
close(fd[0]); // close read end
while (1) {
sprintf(msg, "I send message %d times.", x++);
write(fd[1], msg, MSG_SIZE);
sleep(1);
}
} else { // parent process
pid2 = fork();
if (pid2 == -1) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid2 == 0) { // child process 2
close(fd[1]); // close write end
while (1) {
read(fd[0], msg, MSG_SIZE);
printf("%s\n", msg);
}
} else { // parent process
signal(SIGINT, SIG_IGN); // ignore SIGINT
signal(SIGINT, SIG_DFL); // reset SIGINT to default
signal(SIGINT, SIG_IGN); // ignore SIGINT again
kill(pid1, SIGINT);
kill(pid2, SIGINT);
waitpid(pid1, NULL, 0);
waitpid(pid2, NULL, 0);
close(fd[0]);
close(fd[1]);
printf("Parent Process is Killed!\n");
exit(EXIT_SUCCESS);
}
}
}
```
对于第二个问题,以下是可能的解决方案:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main() {
char *args[] = {"/bin/ls", "-l", NULL};
execv(args[0], args);
perror("execv");
exit(EXIT_FAILURE);
}
```
这个程序使用 `execv` 函数来启动 `/bin/ls` 命令,并传递 `-l` 参数。如果 `execv` 函数返回,说明启动失败,程序将输出错误信息并退出。
相关推荐
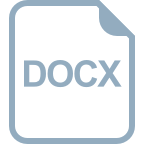
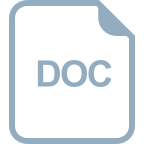
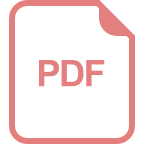













