使用系统调用msgget( ), megsnd( ), msgrev( )及msgctl()编制一长度为1K的消息发送和接收的程序 。
时间: 2023-11-28 12:03:49 浏览: 261
下面是一个使用系统调用 msgget(), msgsnd(), msgrcv() 和 msgctl() 编写的长度为1K的消息发送和接收程序的示例。
发送程序(send.c):
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSGSZ 1024
typedef struct msgbuf {
long mtype;
char mtext[MSGSZ];
} message_buf;
int main()
{
int msqid;
key_t key;
message_buf sbuf;
size_t buflen;
/* 生成一个key */
if ((key = ftok("send.c", 'B')) == -1) {
perror("ftok");
exit(1);
}
/* 连接消息队列 */
if ((msqid = msgget(key, 0644 | IPC_CREAT)) == -1) {
perror("msgget");
exit(1);
}
/* 填充消息结构体 */
sbuf.mtype = 1;
strcpy(sbuf.mtext, "This is a test message.");
buflen = strlen(sbuf.mtext) + 1;
/* 发送消息 */
if (msgsnd(msqid, &sbuf, buflen, 0) == -1) {
perror("msgsnd");
exit(1);
}
printf("Message sent: %s\n", sbuf.mtext);
exit(0);
}
```
接收程序(recv.c):
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSGSZ 1024
typedef struct msgbuf {
long mtype;
char mtext[MSGSZ];
} message_buf;
int main()
{
int msqid;
key_t key;
message_buf rbuf;
/* 生成一个key */
if ((key = ftok("send.c", 'B')) == -1) {
perror("ftok");
exit(1);
}
/* 连接消息队列 */
if ((msqid = msgget(key, 0644)) == -1) {
perror("msgget");
exit(1);
}
/* 接收消息 */
if (msgrcv(msqid, &rbuf, MSGSZ, 1, 0) == -1) {
perror("msgrcv");
exit(1);
}
printf("Message received: %s\n", rbuf.mtext);
/* 删除消息队列 */
if (msgctl(msqid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
exit(0);
}
```
使用方法:
1. 编译发送程序和接收程序:
```
gcc send.c -o send
gcc recv.c -o recv
```
2. 运行发送程序:
```
./send
```
3. 运行接收程序:
```
./recv
```
4. 查看接收程序输出的消息是否与发送程序发送的消息一致。
阅读全文
相关推荐
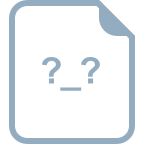
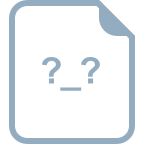







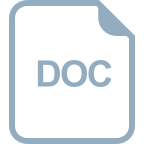
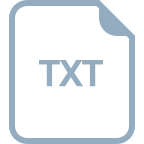
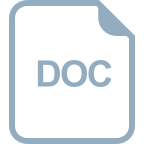
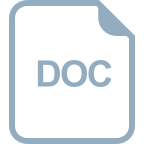
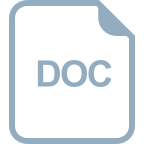
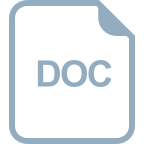