def value(self, x: np.ndarray) -> np.ndarray: ''' Parameters: x is a two dimensional numpy array. Returns: a two dimensioal array representing the element-wise sigmoid of x. ''' #### write your code below #### return 。请帮我完成需要填写的代码
时间: 2024-02-10 09:17:35 浏览: 51
可以使用numpy的sigmoid函数来实现这个方法:
```python
def value(self, x: np.ndarray) -> np.ndarray:
'''
Parameters:
x is a two dimensional numpy array.
Returns:
a two dimensioal array representing the element-wise sigmoid of x.
'''
return 1 / (1 + np.exp(-x))
```
这个方法会返回一个与输入矩阵x相同大小的矩阵,其中每个元素都是sigmoid函数作用于相应的输入元素的结果。
相关问题
def value(self, x: np.ndarray) -> np.ndarray: ''' Parameters: x is a two dimensional numpy array. Returns: a two dimensioal array representing the element-wise sigmoid of x. ''' #### write your code below ####
根据这段代码的要求,我们需要在 `value` 方法中编写代码,实现对输入的二维 numpy 数组 `x` 进行 sigmoid 转换,并返回结果。以下是按照要求编写的代码:
```python
import numpy as np
class MyModel:
def value(self, x: np.ndarray) -> np.ndarray:
"""
Parameters:
x: a two dimensional numpy array
Returns:
a two dimensional array representing the element-wise sigmoid of x.
"""
return 1 / (1 + np.exp(-x))
```
该代码定义了一个名为 `MyModel` 的类,其中包含了一个名为 `value` 的方法,它接收一个二维 numpy 数组 `x` 作为参数,并返回一个二维数组,表示 `x` 中每个元素的 sigmoid 值。
在方法中,我们使用了 numpy 中的 `exp` 函数来计算 $e^{-x}$,然后将其加上 1,并取倒数即可得到 sigmoid 值。
class Softmax(Activation): ''' softmax nonlinear function. ''' def __init__(self): ''' There are no parameters in softmax function. ''' super(Softmax, self).__init__() def value(self, x: np.ndarray) -> np.ndarray: ''' Parameters: x is the input to the softmax function. x is a two dimensional numpy array. Each row is the input to the softmax function Returns: output of the softmax function. The returned value is with the same shape as that of x. ''' #### write your code below #### return def derivative(self, x: np.ndarray) -> np.ndarray: ''' Parameters: x is the input to the softmax function. x is a two dimensional numpy array. Returns: a two dimensional array representing the derivative of softmax function w.r.t. x. ''' #### write your code below ####
下面是对 `Softmax` 激活函数的实现,你可以将其加入到代码中:
```python
class Softmax(Activation):
'''
softmax nonlinear function.
'''
def __init__(self):
'''
There are no parameters in softmax function.
'''
super(Softmax, self).__init__()
def value(self, x: np.ndarray) -> np.ndarray:
'''
Parameters:
x is the input to the softmax function. x is a two dimensional numpy array. Each row is the input to the softmax function
Returns:
output of the softmax function. The returned value is with the same shape as that of x.
'''
exp_scores = np.exp(x)
return exp_scores / np.sum(exp_scores, axis=1, keepdims=True)
def derivative(self, x: np.ndarray) -> np.ndarray:
'''
Parameters:
x is the input to the softmax function. x is a two dimensional numpy array.
Returns:
a two dimensional array representing the derivative of softmax function w.r.t. x.
'''
softmax_output = self.value(x)
return softmax_output * (1 - softmax_output)
```
阅读全文
相关推荐
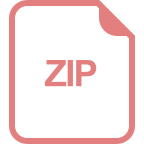














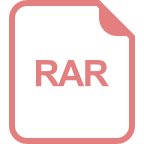