easyx和c++制作反弹球消方块的要求
时间: 2023-11-17 14:04:40 浏览: 174
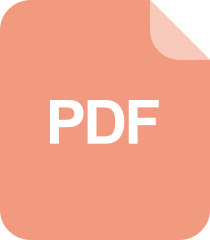
C语言实现反弹球游戏
反弹球游戏和消方块游戏都是比较经典的小游戏,使用easyx库可以很方便地实现这两个游戏。下面是反弹球游戏和消方块游戏的要求:
反弹球游戏要求:
1. 画出一个小球和一个挡板;
2. 小球从挡板上方开始运动,碰到边界会反弹;
3. 小球碰到挡板会反弹,如果小球落到挡板下方,则游戏结束;
4. 挡板可以左右移动,通过键盘控制;
5. 当小球碰到砖块时,砖块会消失,小球会反弹;
6. 当所有砖块都被消除时,游戏胜利。
消方块游戏要求:
1. 画出一个矩形区域,里面有多个小矩形组成的砖块;
2. 有一个小球从上方开始运动,碰到边界会反弹;
3. 有一个挡板可以左右移动,通过键盘控制;
4. 当小球碰到砖块时,砖块会消失,小球会反弹;
5. 当所有砖块都被消除时,游戏胜利;
6. 如果小球落到挡板下方,则游戏结束。
下面是反弹球游戏的代码示例:
```cpp
#include <graphics.h>
#include <conio.h>
#include <time.h>
#include <stdlib.h>
#define BALL_RADIUS 10
#define BLOCK_WIDTH 50
#define BLOCK_HEIGHT 20
#define BLOCK_ROWS 5
#define BLOCK_COLS 10
#define BOARD_WIDTH 100
#define BOARD_HEIGHT 10
#define WINDOW_WIDTH 640
#define WINDOW_HEIGHT 480
int blocks[BLOCK_ROWS][BLOCK_COLS] = {0};
int score = 0;
void initBlocks()
{
for (int i = 0; i < BLOCK_ROWS; i++)
{
for (int j = 0; j < BLOCK_COLS; j++)
{
blocks[i][j] = 1;
}
}
}
void drawBlocks()
{
for (int i = 0; i < BLOCK_ROWS; i++)
{
for (int j = 0; j < BLOCK_COLS; j++)
{
if (blocks[i][j] == 1)
{
int x = j * BLOCK_WIDTH;
int y = i * BLOCK_HEIGHT; setfillcolor(RGB(255, 0, 0));
fillrectangle(x, y, x + BLOCK_WIDTH, y + BLOCK_HEIGHT);
}
}
}
}
void drawBoard(int x)
{
setfillcolor(RGB(0, 255, 0));
fillrectangle(x, WINDOW_HEIGHT - BOARD_HEIGHT, x + BOARD_WIDTH, WINDOW_HEIGHT);
}
void drawBall(int x, int y)
{
setfillcolor(RGB(0, 0, 255));
fillellipse(x, y, BALL_RADIUS, BALL_RADIUS);
}
bool isCollide(int x, int y)
{
if (y + BALL_RADIUS >= WINDOW_HEIGHT - BOARD_HEIGHT && y + BALL_RADIUS <= WINDOW_HEIGHT)
{
if (x + BALL_RADIUS >= boardX && x - BALL_RADIUS <= boardX + BOARD_WIDTH)
{
return true;
}
}
return false;
}
bool isCollideBlock(int x, int y, int &row, int &col)
{
row = (y - BALL_RADIUS) / BLOCK_HEIGHT;
col = x / BLOCK_WIDTH;
if (row >= 0 && row < BLOCK_ROWS && col >= 0 && col < BLOCK_COLS && blocks[row][col] == 1)
{
return true;
}
return false;
}
void updateBall(int &x, int &y, int &vx, int &vy)
{
x += vx;
y += vy;
if (x - BALL_RADIUS <= 0 || x + BALL_RADIUS >= WINDOW_WIDTH)
{
vx = -vx;
}
if (y - BALL_RADIUS <= 0)
{
vy = -vy;
}
if (y + BALL_RADIUS >= WINDOW_HEIGHT)
{
exit(0);
}
if (isCollide(x, y))
{
vy = -vy;
}
int row, col;
if (isCollideBlock(x, y, row, col))
{
blocks[row][col] = 0;
score++;
vy = -vy;
}
}
int main()
{
initgraph(WINDOW_WIDTH, WINDOW_HEIGHT);
initBlocks();
int ballX = WINDOW_WIDTH / 2;
int ballY = WINDOW_HEIGHT / 2;
int ballVX = 5;
int ballVY = 5;
int boardX = WINDOW_WIDTH / 2 - BOARD_WIDTH / 2;
while (true)
{
cleardevice();
drawBlocks();
drawBoard(boardX);
drawBall(ballX, ballY);
updateBall(ballX, ballY, ballVX, ballVY);
if (score == BLOCK_ROWS * BLOCK_COLS)
{
MessageBox(NULL, TEXT("You Win!"), TEXT("Message"), MB_OK);
break;
}
if (_kbhit())
{
int key = _getch();
if (key == 'a' && boardX > 0)
{
boardX -= 10;
}
if (key == 'd' && boardX + BOARD_WIDTH < WINDOW_WIDTH)
{
boardX += 10;
}
}
Sleep(20);
}
closegraph();
return 0;
}
```
下面是消方块游戏的代码示例:
```cpp
#include <graphics.h>
#include <conio.h>
#include <time.h>
#include <stdlib.h>
#define BALL_RADIUS 10
#define BLOCK_WIDTH 50
#define BLOCK_HEIGHT 20
#define BLOCK_ROWS 5
#define BLOCK_COLS 10
#define BOARD_WIDTH 100
#define BOARD_HEIGHT 10
#define WINDOW_WIDTH 640
#define WINDOW_HEIGHT 480
int blocks[BLOCK_ROWS][BLOCK_COLS] = {0};
int score = 0;
void initBlocks()
{
for (int i = 0; i < BLOCK_ROWS; i++)
{
for (int j = 0; j < BLOCK_COLS; j++)
{
blocks[i][j] = rand() % 2;
}
}
}
void drawBlocks()
{
for (int i = 0; i < BLOCK_ROWS; i++)
{
for (int j = 0; j < BLOCK_COLS; j++)
{
if (blocks[i][j] == 1)
{
int x = j * BLOCK_WIDTH;
int y = i * BLOCK_HEIGHT;
setfillcolor(RGB(255, 0, 0));
fillrectangle(x, y, x + BLOCK_WIDTH, y + BLOCK_HEIGHT);
}
}
}
}
void drawBoard(int x)
{
setfillcolor(RGB(0, 255, 0));
fillrectangle(x, WINDOW_HEIGHT - BOARD_HEIGHT, x + BOARD_WIDTH, WINDOW_HEIGHT);
}
void drawBall(int x, int y)
{
setfillcolor(RGB(0, 0, 255));
fillellipse(x, y, BALL_RADIUS, BALL_RADIUS);
}
bool isCollide(int x, int y)
{
if (y + BALL_RADIUS >= WINDOW_HEIGHT - BOARD_HEIGHT && y + BALL_RADIUS <= WINDOW_HEIGHT)
{
if (x + BALL_RADIUS >= boardX && x - BALL_RADIUS <= boardX + BOARD_WIDTH)
{
return true;
}
}
return false;
}
bool isCollideBlock(int x, int y, int &row, int &col)
{
row = (y - BALL_RADIUS) / BLOCK_HEIGHT;
col = x / BLOCK_WIDTH;
if (row >= 0 && row < BLOCK_ROWS && col >= 0 && col < BLOCK_COLS && blocks[row][col] == 1)
{
return true;
}
return false;
}
void updateBall(int &x, int &y, int &vx, int &vy)
{
x += vx;
y += vy;
if (x - BALL_RADIUS <= 0 || x + BALL_RADIUS >= WINDOW_WIDTH)
{
vx = -vx;
}
if (y - BALL_RADIUS <= 0)
{
vy = -vy;
}
if (y + BALL_RADIUS >= WINDOW_HEIGHT)
{
exit(0);
}
if (isCollide(x, y))
{
vy = -vy;
}
int row, col;
if (isCollideBlock(x, y, row, col))
{
blocks[row][col] = 0;
score++;
vy = -vy;
}
}
int main()
{
initgraph(WINDOW_WIDTH, WINDOW_HEIGHT);
srand(time(NULL));
initBlocks();
int ballX = WINDOW_WIDTH / 2;
int ballY = WINDOW_HEIGHT / 2;
int ballVX = 5;
int ballVY = 5;
int boardX = WINDOW_WIDTH / 2 - BOARD_WIDTH / 2;
while (true)
{
cleardevice();
drawBlocks();
drawBoard(boardX);
drawBall(ballX, ballY);
updateBall(ballX, ballY, ballVX, ballVY);
if (score == BLOCK_ROWS * BLOCK_COLS)
{
MessageBox(NULL, TEXT("You Win!"), TEXT("Message"), MB_OK);
break;
}
if (_kbhit())
{
int key = _getch();
if (key == 'a' && boardX > 0)
{
boardX -= 10;
}
if (key == 'd' && boardX + BOARD_WIDTH < WINDOW_WIDTH)
{
boardX += 10;
}
}
Sleep(20);
}
closegraph();
return 0;
}
```
阅读全文
相关推荐
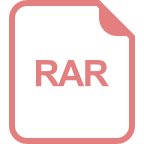
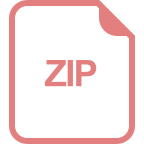
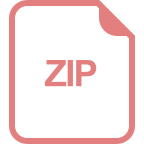
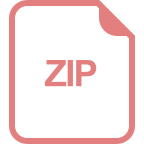
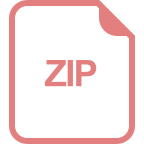
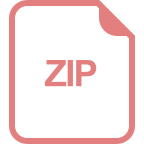
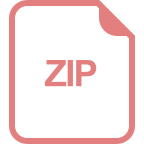
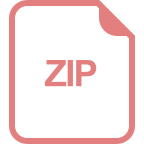
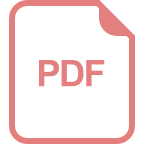
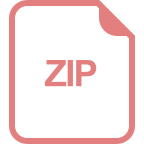
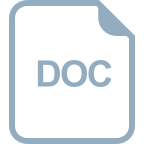




