用代码如何完成二叉树的存储结构的实现及其应用
时间: 2023-12-10 19:39:11 浏览: 86
二叉树的存储结构有两种方式,分别为顺序存储和链式存储。
1. 顺序存储
二叉树的顺序存储方式就是使用一个数组来存储二叉树,数组中的元素表示树的节点,根据数组下标来确定节点之间的关系。对于一个节点 i,它的左子节点为 2i,右子节点为 2i+1,父节点为 i/2。如果某个节点没有子节点,则将对应位置赋值为 null。
下面是使用 Python 实现的二叉树顺序存储结构:
```python
class SeqBinaryTree:
def __init__(self, max_size):
self.max_size = max_size
self.tree = [None] * (max_size + 1)
def add_node(self, value):
if len(self.tree) > self.max_size:
print("Tree is full")
return False
self.tree[len(self.tree)] = value
return True
```
2. 链式存储
二叉树的链式存储方式就是使用一个节点类来表示树的节点,每个节点包括一个值和两个指针分别指向左子节点和右子节点。使用链式存储时,需要定义一个根节点,它指向整个二叉树的根节点。
下面是使用 Python 实现的二叉树链式存储结构:
```python
class TreeNode:
def __init__(self, value):
self.value = value
self.left_child = None
self.right_child = None
class BinaryTree:
def __init__(self):
self.root = None
def add_node(self, value):
node = TreeNode(value)
if self.root is None:
self.root = node
else:
queue = [self.root]
while queue:
curr = queue.pop(0)
if curr.left_child is None:
curr.left_child = node
return
elif curr.right_child is None:
curr.right_child = node
return
else:
queue.append(curr.left_child)
queue.append(curr.right_child)
```
应用:
二叉树的存储结构可以用于二叉树的遍历和搜索。例如,使用深度优先遍历算法(前序遍历、中序遍历、后序遍历)可以对二叉树进行遍历;使用广度优先遍历算法(层序遍历)可以对二叉树进行搜索。此外,二叉树的存储结构还可以用于实现堆、哈夫曼树等数据结构。
阅读全文
相关推荐
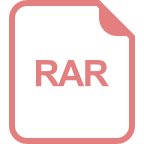
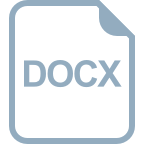
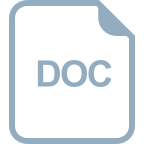
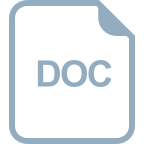
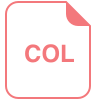
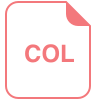
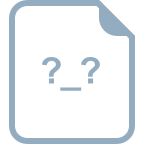
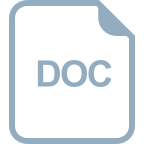
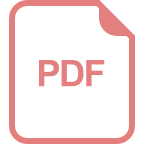
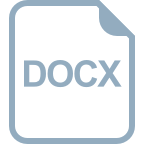
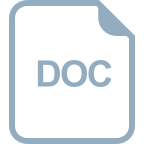
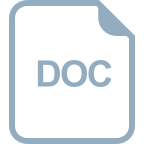
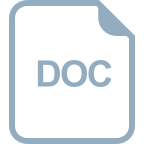
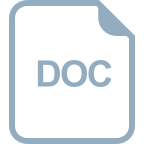
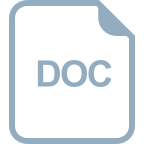