使用python实现:通过输入行权价格和股票价格计算期权的希腊字母并且绘制波动率微笑图形
时间: 2023-08-11 07:04:08 浏览: 165
要计算期权的希腊字母,需要使用Black-Scholes模型或其他期权定价模型。这里我们使用Black-Scholes模型来计算希腊字母,并使用matplotlib库绘制波动率微笑曲线。
首先,需要安装scipy和matplotlib库。可以使用以下命令进行安装:
```
pip install scipy matplotlib
```
接下来,可以使用以下代码实现期权希腊字母的计算和波动率微笑曲线的绘制:
```python
import numpy as np
from scipy.stats import norm
import matplotlib.pyplot as plt
def bs_price(S, K, T, r, sigma, option='call'):
"""
计算期权价格
"""
d1 = (np.log(S / K) + (r + 0.5 * sigma ** 2) * T) / (sigma * np.sqrt(T))
d2 = d1 - sigma * np.sqrt(T)
if option == 'call':
return S * norm.cdf(d1) - K * np.exp(-r * T) * norm.cdf(d2)
else:
return K * np.exp(-r * T) * norm.cdf(-d2) - S * norm.cdf(-d1)
def bs_delta(S, K, T, r, sigma, option='call'):
"""
计算Delta
"""
d1 = (np.log(S / K) + (r + 0.5 * sigma ** 2) * T) / (sigma * np.sqrt(T))
if option == 'call':
return norm.cdf(d1)
else:
return -norm.cdf(-d1)
def bs_gamma(S, K, T, r, sigma):
"""
计算Gamma
"""
d1 = (np.log(S / K) + (r + 0.5 * sigma ** 2) * T) / (sigma * np.sqrt(T))
return norm.pdf(d1) / (S * sigma * np.sqrt(T))
def bs_vega(S, K, T, r, sigma):
"""
计算Vega
"""
d1 = (np.log(S / K) + (r + 0.5 * sigma ** 2) * T) / (sigma * np.sqrt(T))
return S * norm.pdf(d1) * np.sqrt(T)
def smile(S, K, T, r, vols):
"""
绘制波动率微笑曲线
"""
call_vols = []
put_vols = []
for vol in vols:
call_vols.append(bs_price(S, K, T, r, vol))
put_vols.append(bs_price(S, K, T, r, vol, option='put'))
plt.plot(K, call_vols, 'r', label='Call')
plt.plot(K, put_vols, 'b', label='Put')
plt.xlabel('Strike Price')
plt.ylabel('Option Price')
plt.title('Volatility Smile')
plt.legend()
plt.show()
# 示例
S = 100 # 股票价格
K = np.linspace(80, 120, 41) # 行权价格
T = 1 # 看涨期权到期时间
r = 0.05 # 无风险利率
vols = np.linspace(0.1, 0.5, 9) # 波动率
smile(S, K, T, r, vols)
```
在上面的代码中,我们定义了bs_price、bs_delta、bs_gamma和bs_vega函数分别计算期权价格、Delta、Gamma和Vega。然后,我们定义了smile函数来绘制波动率微笑曲线。最后,我们使用示例数据调用smile函数并绘制波动率微笑曲线。
阅读全文
相关推荐
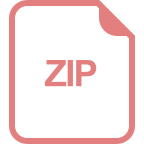
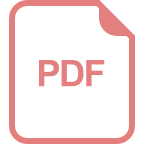


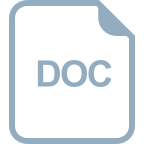





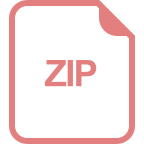
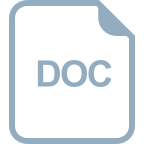
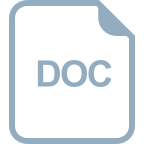
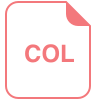
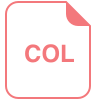
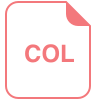
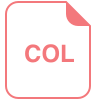
