sequential中加入attention层
时间: 2023-07-29 09:06:18 浏览: 189
在使用sequential构建神经网络时,可以通过添加keras.layers.Attention层来实现注意力机制。例如:
```
from tensorflow.keras.layers import Dense, Input, Embedding, LSTM, Attention
from tensorflow.keras.models import Model, Sequential
# 定义模型
model = Sequential()
model.add(Embedding(input_dim=vocab_size, output_dim=embedding_dim, input_length=max_len))
model.add(LSTM(units=hidden_units, return_sequences=True))
model.add(Attention())
model.add(Dense(units=output_dim, activation='softmax'))
# 编译模型
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
```
在上述代码中,我们在LSTM层后面添加了Attention层,并且在Dense层前面使用了softmax激活函数,完成了一个简单的带有注意力机制的序列分类模型。
相关问题
model=Sequential()中加入attention
在使用Keras构建神经网络时,可以通过在Sequential模型中添加Attention层来实现注意力机制。
首先,需要导入相关的库:
```
from keras.layers import Input, Dense, LSTM, concatenate, Activation, Masking
from keras.models import Model
from keras import backend as K
```
接下来,创建一个Attention层的类:
```
class AttentionLayer(Layer):
def __init__(self, **kwargs):
super(AttentionLayer, self).__init__(**kwargs)
def build(self, input_shape):
self.W = self.add_weight(name='att_weight', shape=(input_shape[-1], 1), initializer='normal')
self.b = self.add_weight(name='att_bias', shape=(input_shape[1], 1), initializer='zeros')
super(AttentionLayer, self).build(input_shape)
def call(self, x):
e = K.tanh(K.dot(x, self.W) + self.b)
a = K.softmax(e, axis=1)
output = x * a
return K.sum(output, axis=1)
def compute_output_shape(self, input_shape):
return (input_shape[0], input_shape[-1])
```
该类继承自Keras的Layer类,重写了build和call方法。在build方法中初始化权重矩阵W和偏置向量b,利用Keras的add_weight方法实现。在call方法中,计算出每个时间步的注意力权重a,对输入进行加权求和并返回。
最后,在Sequential模型中加入Attention层:
```
model = Sequential()
model.add(LSTM(128, input_shape=(20, 100), return_sequences=True))
model.add(AttentionLayer())
model.add(Dense(64, activation='relu'))
model.add(Dense(1, activation='sigmoid'))
```
这里以LSTM作为示例,先在LSTM层后加入Attention层,再通过Dense层输出结果。注意,Attention层需要在return_sequences=True时使用。
RegGAN加入attention代码
好的,以下是一个简单的示例,展示如何将attention机制加入到RegGAN中:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class RegGAN(nn.Module):
def __init__(self, input_size, output_size, hidden_size, num_layers, dropout, attention_size):
super(RegGAN, self).__init__()
# 定义生成器
self.generator = nn.Sequential(
nn.Linear(input_size, hidden_size),
nn.LeakyReLU(0.2),
nn.Dropout(dropout),
nn.Linear(hidden_size, hidden_size),
nn.LeakyReLU(0.2),
nn.Dropout(dropout),
nn.Linear(hidden_size, output_size),
nn.Tanh()
)
# 定义鉴别器
self.discriminator = nn.Sequential(
nn.Linear(output_size, hidden_size),
nn.LeakyReLU(0.2),
nn.Linear(hidden_size, hidden_size),
nn.LeakyReLU(0.2),
nn.Linear(hidden_size, 1),
nn.Sigmoid()
)
# 定义attention机制
self.attention = nn.Sequential(
nn.Linear(hidden_size, attention_size),
nn.Tanh(),
nn.Linear(attention_size, 1),
nn.Softmax(dim=1)
)
def forward(self, x):
# 生成器前向传播
x_gen = self.generator(x)
# 鉴别器前向传播
d_out = self.discriminator(x_gen)
# 计算attention权重
attn_weights = self.attention(x_gen)
# 将attention权重与生成器输出相乘
x_out = (attn_weights * x_gen).sum(dim=1)
return x_out, d_out
```
在上面的代码中,我们添加了一个名为`attention`的新神经网络层,它接受生成器的输出作为输入,并输出一个权重向量。我们使用一个简单的两层全连接神经网络来实现attention机制,其中第一层将生成器输出映射到一个较小的维度,第二层将其映射到一个标量。最后,我们使用softmax函数将这个标量转换为一个权重向量,该向量的每个元素表示相应生成器输出的相对重要性。
然后,在`forward`函数中,我们首先通过生成器生成一批数据,然后将其传递给鉴别器。接下来,我们将生成器的输出传递给attention层,获取attention权重。最后,我们将attention权重与生成器的输出相乘,并对结果进行求和,以得到最终的输出。
相关推荐
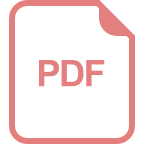
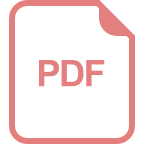
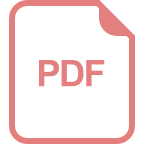













