用C++语言设计一个图形基类:Shape,在此基础上派生Rectangle、Circle、Triangle 类。 1、Cirlce 类基本信息:圆心坐标、半径; Rectangle 类基本信息:长、宽; Triangle 类基本信息:三个顶点坐标; 其中:成员变量为 private 属性,成员函数为public 属性; 2、每个图形类有多个构造函数:缺省构造函数、带参数的构造函数; 3、每个图形类有计算图形的面积GetArea(),显示图形的基本信息函数Show(),修改基 本信息的函数Set(形参)。 [实验提示] 1、在派生类的构造函数中,应注意对基类数据成员的初始化; 2、各类图形面积的计算方法不同, 因此不能在基类 Shape 中统一确定计算的方法。在 基类Shape 中实现计算面积功能的函数体GetArea()应为空,在派生类中根据同名覆盖原则 定义各自的同名函数实现具体功能
时间: 2024-02-22 07:56:56 浏览: 70
好的,我可以为您解答这个问题。下面是C++代码实现:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Shape {
protected:
double area;
public:
Shape() {};
virtual ~Shape() {};
virtual double GetArea() = 0;
virtual void Show() = 0;
virtual void Set() = 0;
};
class Circle : public Shape {
private:
double x, y, r;
public:
Circle() : x(0), y(0), r(0) {};
Circle(double x, double y, double r) : x(x), y(y), r(r) {};
~Circle() {};
double GetArea() {
area = 3.1415926 * r * r;
return area;
}
void Show() {
cout << "Circle: x=" << x << ", y=" << y << ", r=" << r << ", area=" << area << endl;
}
void Set(double x, double y, double r) {
this->x = x;
this->y = y;
this->r = r;
}
};
class Rectangle : public Shape {
private:
double l, w;
public:
Rectangle() : l(0), w(0) {};
Rectangle(double l, double w) : l(l), w(w) {};
~Rectangle() {};
double GetArea() {
area = l * w;
return area;
}
void Show() {
cout << "Rectangle: l=" << l << ", w=" << w << ", area=" << area << endl;
}
void Set(double l, double w) {
this->l = l;
this->w = w;
}
};
class Triangle : public Shape {
private:
double x1, y1, x2, y2, x3, y3;
public:
Triangle() : x1(0), y1(0), x2(0), y2(0), x3(0), y3(0) {};
Triangle(double x1, double y1, double x2, double y2, double x3, double y3)
: x1(x1), y1(y1), x2(x2), y2(y2), x3(x3), y3(y3) {};
~Triangle() {};
double GetArea() {
double p = (sqrt(pow((x2 - x1), 2) + pow((y2 - y1), 2)) + sqrt(pow((x3 - x2), 2) + pow((y3 - y2), 2))
+ sqrt(pow((x1 - x3), 2) + pow((y1 - y3), 2))) / 2;
area = sqrt(p * (p - sqrt(pow((x2 - x1), 2) + pow((y2 - y1), 2))) * (p - sqrt(pow((x3 - x2), 2) + pow((y3 - y2), 2)))
* (p - sqrt(pow((x1 - x3), 2) + pow((y1 - y3), 2))));
return area;
}
void Show() {
cout << "Triangle: (" << x1 << ", " << y1 << "), (" << x2 << ", " << y2 << "), (" << x3 << ", " << y3 << "), area=" << area << endl;
}
void Set(double x1, double y1, double x2, double y2, double x3, double y3) {
this->x1 = x1;
this->y1 = y1;
this->x2 = x2;
this->y2 = y2;
this->x3 = x3;
this->y3 = y3;
}
};
int main() {
Circle c1(0, 0, 1);
c1.GetArea();
c1.Show();
c1.Set(2, 2, 3);
c1.GetArea();
c1.Show();
Rectangle r1(2, 3);
r1.GetArea();
r1.Show();
r1.Set(4, 5);
r1.GetArea();
r1.Show();
Triangle t1(0, 0, 3, 0, 0, 4);
t1.GetArea();
t1.Show();
t1.Set(0, 0, 6, 0, 0, 8);
t1.GetArea();
t1.Show();
return 0;
}
```
在这个代码中,我们首先定义了一个基类 Shape,其中包含了一个公共的成员函数 GetArea,用于计算图形的面积,以及一个 Show 函数,用于显示图形的基本信息,以及一个 Set 函数,用于修改图形的基本信息。
然后,我们定义了三个派生类 Circle、Rectangle 和 Triangle,它们分别继承了 Shape 基类,并且实现了自己的 GetArea、Show 和 Set 函数,用于计算自己图形的面积,显示自己的基本信息,以及修改自己的基本信息。
最后,在 main 函数中,我们分别创建了几个不同的图形对象,并且调用了它们的 GetArea、Show 和 Set 函数,来展示我们的程序的功能。
相关推荐
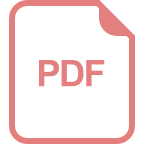
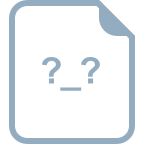
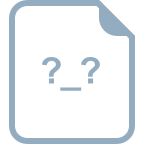














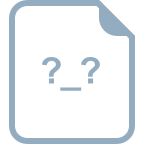