void detectAndDisplay(Mat frame) { Mat frame_gray; cvtColor(frame, frame_gray, COLOR_BGR2GRAY); equalizeHist(frame_gray, frame_gray); //-- Detect faces std::vector<Rect> faces; face_cascade.detectMultiScale(frame_gray, faces); for (size_t i = 0; i < faces.size(); i++) { Point center(faces[i].x + faces[i].width / 2, faces[i].y + faces[i].height / 2); ellipse(frame, center, Size(faces[i].width / 2, faces[i].height / 2), 0, 0, 360, Scalar(255, 0, 255), 4); Mat faceROI = frame_gray(faces[i]); //-- In each face, detect eyes std::vector<Rect> eyes; eyes_cascade.detectMultiScale(faceROI, eyes); for (size_t j = 0; j < eyes.size(); j++) { Point eye_center(faces[i].x + eyes[j].x + eyes[j].width / 2, faces[i].y + eyes[j].y + eyes[j].height / 2); int radius = cvRound((eyes[j].width + eyes[j].height) * 0.25); circle(frame, eye_center, radius, Scalar(255, 0, 0), 4); } } //-- Show what you got imshow("Capture - Face detection", frame); }
时间: 2024-01-28 16:05:42 浏览: 13
这段代码是使用OpenCV库进行人脸和眼部检测的函数。它首先将彩色图像转换为灰度图像,然后通过对灰度图像进行直方图均衡化来增强图像质量。然后使用级联分类器(face_cascade)检测人脸,并在每个检测到的人脸上绘制一个椭圆。对于每个检测到的人脸,它会在其上检测眼睛,并在每个检测到的眼睛上绘制一个圆。最后,它将处理后的图像显示出来。
相关问题
java opencv 调用摄像头_opencv java实现调用摄像头动态识别人脸
好的,以下是使用 Java OpenCV 库实现调用摄像头动态识别人脸的代码示例:
```java
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.core.MatOfRect;
import org.opencv.core.Point;
import org.opencv.core.Rect;
import org.opencv.core.Scalar;
import org.opencv.core.Size;
import org.opencv.imgproc.Imgproc;
import org.opencv.objdetect.CascadeClassifier;
import org.opencv.videoio.VideoCapture;
public class FaceRecognition {
public static void main(String[] args) {
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
CascadeClassifier faceDetector = new CascadeClassifier("haarcascade_frontalface_alt.xml");
VideoCapture capture = new VideoCapture(0);
MatOfRect faceDetections = new MatOfRect();
Mat frame = new Mat();
while (capture.read(frame)) {
Imgproc.cvtColor(frame, frame, Imgproc.COLOR_BGR2GRAY);
Imgproc.equalizeHist(frame, frame);
faceDetector.detectMultiScale(frame, faceDetections, 1.1, 3, 0, new Size(30, 30), new Size());
for (Rect rect : faceDetections.toArray()) {
Imgproc.rectangle(frame, new Point(rect.x, rect.y), new Point(rect.x + rect.width, rect.y + rect.height), new Scalar(0, 255, 0), 2);
}
HighGui.imshow("Face Detection", frame);
if (HighGui.waitKey(1) == 27) {
break;
}
}
capture.release();
HighGui.destroyAllWindows();
}
}
```
代码中使用了 OpenCV 自带的人脸检测分类器 `haarcascade_frontalface_alt.xml` 进行人脸检测。通过 `VideoCapture(0)` 调用摄像头进行图像采集,并使用 `while` 循环处理采集到的每一帧图像。
在每一帧图像中,首先将图像转换为灰度图像,并使用直方图均衡化处理,以增强人脸检测的效果。然后使用 `faceDetector.detectMultiScale()` 方法进行人脸检测,返回一个 `MatOfRect` 类型的结果,其中包含检测到的所有人脸的矩形框。
最后,遍历每个矩形框,并使用 `Imgproc.rectangle()` 方法在原图像中画出矩形框,以显示检测结果。同时使用 `HighGui.imshow()` 方法将处理后的图像显示在窗口中,并使用 `HighGui.waitKey()` 方法等待用户按下 ESC 键结束程序。
希望这段代码能对你有所帮助。
java opencv 调用摄像头_opencv java实现调用摄像头文字识别
好的,要使用Java OpenCV调用摄像头并进行文字识别,您需要遵循以下步骤:
1. 安装Tesseract OCR引擎
Tesseract OCR引擎是一个开源的OCR引擎,可以识别多种语言的文本。您可以从Tesseract OCR官方网站下载并安装Tesseract OCR引擎。
2. 导入Tesseract OCR库
在Java项目中,您需要导入Tesseract OCR库。您可以使用Maven和Gradle等构建工具来导入Tesseract OCR库。
3. 调用摄像头
您可以使用Java OpenCV中的VideoCapture类调用摄像头。以下是一个简单的示例代码:
```
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.videoio.VideoCapture;
public class CameraCapture {
public static void main(String[] args) {
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
VideoCapture camera = new VideoCapture(0);
if(!camera.isOpened()){
System.out.println("Error");
}
else {
Mat frame = new Mat();
while(true){
if (camera.read(frame)){
System.out.println("Frame Obtained");
System.out.println("Captured Frame Width " +
frame.width() + " Height " + frame.height());
Core.flip(frame, frame, 1);
break;
}
}
}
camera.release();
}
}
```
此代码段将获取从摄像头获取的帧并将其翻转。
4. 文字识别
要进行文字识别,您可以使用Java OpenCV中的Imgcodecs类将帧转换为图像,然后使用Tesseract OCR库进行识别。以下是一个简单的示例代码:
```
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.videoio.VideoCapture;
import net.sourceforge.tess4j.*;
public class TextRecognition {
public static void main(String[] args) {
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
VideoCapture camera = new VideoCapture(0);
Mat frame = new Mat();
while (true){
if (camera.read(frame)){
Mat grayFrame = new Mat();
Imgproc.cvtColor(frame, grayFrame, Imgproc.COLOR_BGR2GRAY);
File imageFile = new File("image.png");
Imgcodecs.imwrite(imageFile.getAbsolutePath(), grayFrame);
ITesseract tess = new Tesseract();
tess.setDatapath("tessdata");
tess.setLanguage("eng");
String result = tess.doOCR(imageFile);
System.out.println(result);
HighGui.imshow("Text Recognition", frame);
HighGui.waitKey(1);
}
}
}
}
```
此代码段将在从摄像头获取的帧中识别文本。它首先将帧转换为灰度图像,然后将其保存到磁盘上的图像文件中。然后,它使用Tesseract OCR库对图像文件中的文本进行识别,并将结果打印到控制台上。您需要将Tesseract OCR库的数据路径设置为“tessdata”文件夹,其中包含识别语言的数据文件。
希望这可以帮助您开始使用Java OpenCV调用摄像头并进行文字识别。
相关推荐
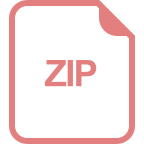
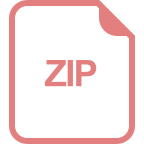












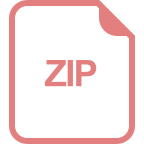