用C++完成以下任务:有四个圆塔,圆心分别为(2,2)、(-2,2)、(-2,-2)、(2,-2),圆半径为1.这4个塔的高度为 10m,塔以下无建筑物。今输入任一点的坐标,求该点的建筑高度(塔外高度为O)
时间: 2024-10-24 14:12:53 浏览: 50
要计算给定点的建筑高度,我们首先需要定义一个结构体表示圆塔,然后编写一个函数来检查这个点是否位于任何一个圆内。如果在圆内,返回塔高;如果在圆外,则返回0。
```cpp
#include <cmath>
// 圆塔结构体
struct Tower {
double x, y; // 圆心坐标
double radius; // 半径
int height; // 塔高
};
// 检查点是否在某个圆内
bool isInsideCircle(double x, double y, Tower tower) {
double distance = std::sqrt(std::pow(x - tower.x, 2) + std::pow(y - tower.y, 2));
return distance <= tower.radius;
}
// 计算建筑高度
int getBuildingHeight(double inputX, double inputY, Tower towers[4]) {
for (Tower tower : towers) {
if (isInsideCircle(inputX, inputY, tower)) {
return tower.height;
}
}
return 0; // 如果不在任何圆内,建筑高度为0
}
int main() {
Tower towers[] = { {2, 2, 1, 10}, {-2, 2, 1, 10}, {-2, -2, 1, 10}, {2, -2, 1, 10} };
double inputX, inputY;
// 用户输入点的坐标
std::cout << "Enter the coordinates (x, y): ";
std::cin >> inputX >> inputY;
int buildingHeight = getBuildingHeight(inputX, inputY, towers);
std::cout << "The building height at the given point (" << inputX << ", " << inputY << ") is: " << buildingHeight << " meters." << std::endl;
return 0;
}
```
在这个程序中,用户会被提示输入点的坐标(x, y),然后`getBuildingHeight`函数会根据这些信息判断点的位置并返回相应的建筑高度。如果输入的点在所有塔之外,输出将是0米。
阅读全文
相关推荐
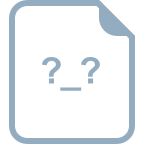
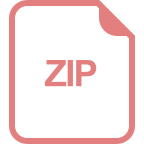
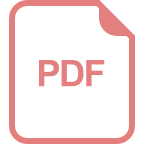















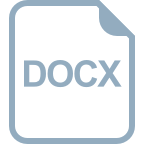