Write a simple GUI program with a button that responds to events from a mouse being pressed and released. The button should display: • the message “No action” when no action is taken on the mouse. • the message “Pressing down” when the mouse is pressed.
时间: 2023-11-22 11:56:54 浏览: 32
Here's an example program using Python and the tkinter library to create a simple GUI with a button that responds to mouse events:
```
import tkinter as tk
class MouseButtonApp:
def __init__(self, master):
self.master = master
master.title("Mouse Button App")
self.button = tk.Button(master, text="No action", command=self.no_action)
self.button.pack()
self.button.bind("<ButtonPress-1>", self.press_down)
self.button.bind("<ButtonRelease-1>", self.release_up)
def no_action(self):
self.button.configure(text="No action")
def press_down(self, event):
self.button.configure(text="Pressing down")
def release_up(self, event):
self.button.configure(text="No action")
if __name__ == '__main__':
root = tk.Tk()
app = MouseButtonApp(root)
root.mainloop()
```
When the program is run, it will create a window with a button that displays "No action". When the button is pressed, it will change the text to "Pressing down", and when the button is released, it will change the text back to "No action".
相关推荐
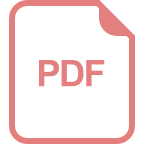
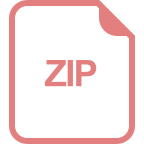
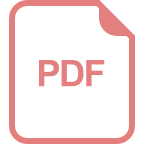














