下面的程序将打印出什么?解释原因 #include <stdio.h> int main(void) { int ref[ ] = {8, 4, 0, 2}; int *ptr; int index; for(index = 0, ptr = ref; index<4; index++, ptr++) printf(“%d %d\n”, ref[index], *ptr); return 0; }
时间: 2024-04-08 08:35:25 浏览: 121
该程序将打印出以下内容:
```
8 8
4 4
0 0
2 2
```
解释原因:
在该程序中,定义了一个整型数组ref,并初始化为{8, 4, 0, 2}。接着定义了一个整型指针ptr和一个整型变量index。在for循环中,初始时index为0,ptr指向数组ref的第一个元素。
在循环体内部,通过ref[index]访问数组ref中的元素,并通过*ptr访问指针ptr所指向的元素。由于ptr一开始指向ref的第一个元素,因此ref[index]和*ptr都会得到相同的值。
随着循环的进行,index递增,ptr也递增指向下一个元素,所以每次循环都会打印出相同的两个值。
因此,程序会打印出4对相同的数字,分别是8、4、0和2。
相关问题
include<stdio.h> int main() { int x; scanf("%d"&x); if(x/3==0&&x/5==0) } printf("%d"x); return;
`include<stdio.h>` 是 C 语言中的预处理器指令,用于包含标准输入输出库(stdio)的功能,如 `scanf()` 和 `printf()` 函数。在您的代码片段中,它引入了标准 I/O 库以便于进行输入和输出操作。
这是您提供的 C 代码:
```csharp
using System;
class Program
{
static void Main()
{
int x;
scanf("%d", ref x); // 注意这里使用 "ref" 关键字,它是 C# 中的传引用参数而不是 C 的 "&"
if (x % 3 == 0 && x % 5 == 0)
{
Console.WriteLine(x); // C# 使用 Console.WriteLine 替代 printf
}
return;
}
}
```
这段代码定义了一个名为 `Main` 的主函数,在其中:
1. 声明了一个整数变量 `x`,并从用户那里读取一个整数值。
2. 使用 `scanf` 或者在 C# 中为 `Console.ReadLine()` 或 `int.TryParse(Console.ReadLine(), out x)` 来获取用户输入。
3. 检查 `x` 是否同时能被 3 和 5 整除,如果满足条件,则打印 `x` 的值。
4. 结束程序。
C 语言里的 `&x` 是地址-of 运算符,而 C# 里用 `ref x` 表示传递变量的地址。此外,C# 使用 `Console.WriteLine` 输出字符串和值,而不是 `%d` 格式化字符串。
hashtable ref
哈希表(Hashtable)是一种数据结构,它可以将键(Key)与值(Value)进行关联存储。在哈希表中,通过一个哈希函数将键映射到一个索引位置,该索引位置对应的存储位置就是与该键关联的值。
在C语言中,可以使用指针和结构体来实现哈希表。具体实现方式可以参考以下代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SIZE 10
// 定义哈希节点结构体
typedef struct Node {
int key;
int value;
struct Node* next;
} Node;
// 创建哈希节点
Node* createNode(int key, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->key = key;
newNode->value = value;
newNode->next = NULL;
return newNode;
}
// 哈希函数
int hashFunction(int key) {
return key % SIZE;
}
// 插入键值对
void insert(Node** hashTable, int key, int value) {
int index = hashFunction(key);
Node* newNode = createNode(key, value);
if (hashTable[index] == NULL) {
hashTable[index] = newNode;
} else {
Node* current = hashTable[index];
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 查找键对应的值
int search(Node** hashTable, int key) {
int index = hashFunction(key);
Node* current = hashTable[index];
while (current != NULL) {
if (current->key == key) {
return current->value;
}
current = current->next;
}
return -1; // 未找到
}
int main() {
Node** hashTable = (Node**)malloc(SIZE * sizeof(Node*));
memset(hashTable, 0, SIZE * sizeof(Node*));
// 插入键值对
insert(hashTable, 1, 10);
insert(hashTable, 2, 20);
insert(hashTable, 3, 30);
// 查找键对应的值
int value = search(hashTable, 2);
if (value != -1) {
printf("Found value: %d\n", value);
} else {
printf("Key not found\n");
}
return 0;
}
```
以上代码实现了一个简单的哈希表,其中使用了哈希函数将键映射到索引位置,并通过链表处理哈希冲突(多个键映射到同一个索引位置)。你可以根据需要自行扩展和优化该实现。
阅读全文
相关推荐
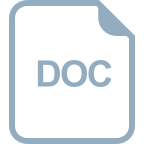
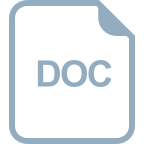
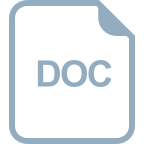













