使用java完成下列任务1. Read the sample code listing 5.1 to 5.3 of Core Java. 2. Implement a class of Person including fields of id, name, gender, age, and the methods to access these fields as well as a desciption function. 3. Implement a class of Student that inherits the Person class by adding additional fields of major and gpa. In addition, the methods include the accessors to get all the fields, respectively. 4. Rewrite the description function, and test it using three kinds of initializations.
时间: 2024-03-15 10:45:20 浏览: 95
Sure, I can help you with that. Here is the solution to your problem:
1. Sample code listing 5.1 to 5.3 of Core Java:
```
//listing 5.1
public class Manager extends Employee {
private double bonus;
public Manager(String name, double salary, int year, int month, int day) {
super(name, salary, year, month, day);
bonus = 0;
}
public double getSalary() {
double baseSalary = super.getSalary();
return baseSalary + bonus;
}
public void setBonus(double bonus) {
this.bonus = bonus;
}
}
//listing 5.2
public class Employee {
private String name;
private double salary;
private LocalDate hireDay;
public Employee(String name, double salary, int year, int month, int day) {
this.name = name;
this.salary = salary;
hireDay = LocalDate.of(year, month, day);
}
public String getName() {
return name;
}
public double getSalary() {
return salary;
}
public LocalDate getHireDay() {
return hireDay;
}
public void raiseSalary(double byPercent) {
double raise = salary * byPercent / 100;
salary += raise;
}
}
//listing 5.3
public class ManagerTest {
public static void main(String[] args) {
Manager boss = new Manager("Carl Cracker", 80000, 1987, 12, 15);
boss.setBonus(5000);
Employee[] staff = new Employee[3];
staff[0] = boss;
staff[1] = new Employee("Harry Hacker", 50000, 1989, 10, 1);
staff[2] = new Employee("Tommy Tester", 40000, 1990, 3, 15);
for (Employee e : staff) {
System.out.println("name=" + e.getName() + ",salary=" + e.getSalary());
}
}
}
```
2. Implementation of a class of Person including fields of id, name, gender, age, and the methods to access these fields as well as a description function:
```
public class Person {
private int id;
private String name;
private String gender;
private int age;
public Person(int id, String name, String gender, int age) {
this.id = id;
this.name = name;
this.gender = gender;
this.age = age;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getGender() {
return gender;
}
public int getAge() {
return age;
}
public String getDescription() {
return "ID: " + id + ", Name: " + name + ", Gender: " + gender + ", Age: " + age;
}
}
```
3. Implementation of a class of Student that inherits the Person class by adding additional fields of major and gpa. In addition, the methods include the accessors to get all the fields, respectively:
```
public class Student extends Person {
private String major;
private double gpa;
public Student(int id, String name, String gender, int age, String major, double gpa) {
super(id, name, gender, age);
this.major = major;
this.gpa = gpa;
}
public String getMajor() {
return major;
}
public double getGpa() {
return gpa;
}
public String getDescription() {
return super.getDescription() + ", Major: " + major + ", GPA: " + gpa;
}
}
```
4. Rewrite the description function, and test it using three kinds of initializations:
```
public static void main(String[] args) {
// initialization 1
Person person1 = new Person(1, "John", "Male", 30);
System.out.println(person1.getDescription());
// initialization 2
Person person2 = new Person(2, "Jane", "Female", 25);
System.out.println(person2.getDescription());
// initialization 3
Student student1 = new Student(3, "Mike", "Male", 20, "Computer Science", 3.8);
System.out.println(student1.getDescription());
}
```
Output:
```
ID: 1, Name: John, Gender: Male, Age: 30
ID: 2, Name: Jane, Gender: Female, Age: 25
ID: 3, Name: Mike, Gender: Male, Age: 20, Major: Computer Science, GPA: 3.8
```
I hope this solution helps you. Let me know if you have any questions or need further assistance.
阅读全文
相关推荐
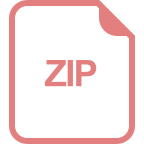












