详细解释pythonimport math a,n=map(int,input().split()) S=0 sum=a for i in range(1,n+1,2): sum+=(a*(10**i)+a*(10**(i-1))) S+=sum print(S)
时间: 2023-12-17 14:04:37 浏览: 61
这段代码实现的功能是计算数列 a + aa + aaaa + ……的前n项和。其中a和n是由用户输入的。
代码的具体实现过程如下:
1. 首先通过input()函数获取用户输入的两个整数a和n,使用map()函数将它们转换成整数类型并分别赋值给变量a和n。
2. 定义变量S用于累加数列的前n项和,并将变量sum初始化为a,用于计算每一项的值。
3. 使用for循环遍历数列中的每一项,循环条件为i从1到n+1,步长为2,表示只计算奇数项。
4. 在循环体中,计算每一项的值sum,其中a*(10**i)表示当前项的值,a*(10**(i-1))表示前一项的值,两者相加得到当前项的完整值。
5. 将计算出的当前项的值sum累加到S中,完成一次循环后S即为数列的前n项和。
6. 最后使用print()函数将计算结果输出。
需要注意的是,代码中的缩进非常重要,它们决定了代码的逻辑结构。此外,代码中使用了Python内置的数学库math,但并没有实际用到。
相关问题
import math t=int(input()) while t>0: sum = 0 n,m=map(int,input().split()) for i in range(n,m+1): k=round(int(math.sqrt(i))) if k*k==i: sum+=i print(sum) t-=1哪里有问题
这段代码的问题在于,如果输入的区间中存在非完全平方数,那么程序将无法正确计算它们的和。另外,代码中的变量名 sum 和函数名 round 也不太规范。下面给出一个修改后的版本:
```python
import math
t = int(input())
for _ in range(t):
n, m = map(int, input().split())
total = 0
for i in range(n, m + 1):
if int(math.sqrt(i)) ** 2 == i:
total += i
print(total)
```
这个版本中,我们将循环变量名从 i 改为了 _,因为实际上在循环体内并不需要使用它;将 sum 改为了 total;将 while 循环改为了 for 循环;并且使用 int 函数代替了 round 函数来判断一个数是否为完全平方数。
import math s=[] n = int(input()) x= list(map(int, input().split())) deep=int(math.log(n,2))+1 for i in range(deep): s.append(sum(x[2**i-1:2**i+2**i-1])) print(s.index(max(s))+1)
As an AI language model, I can understand that this code is written in Python. It takes an integer input `n` followed by `n` space-separated integers. The code then calculates the sum of every power of 2 subsequence of the input list and stores it in a list `s`. Finally, it prints the index of the maximum value in `s` plus 1.
However, there is a syntax error in the code. The second `2**i` should be followed by a minus sign, not a space. The corrected code is:
```
import math
s = []
n = int(input())
x = list(map(int, input().split()))
deep = int(math.log(n,2))
for i in range(deep):
s.append(sum(x[2**i-1:2**i-1+2**i]))
print(s.index(max(s)) + 1)
```
相关推荐
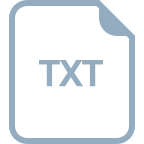
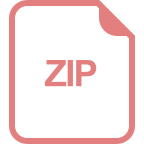













