利用c语言写出伪代码,并写出每一种的做法:试设计四个算法,分别计算: (1)二叉树中结点个数; (2)二叉树的深度; (3)二叉链表树中指定结点的层次数; (4)二叉链表树的叶结点数;(该问为中科院计算所研究生招生试题) (5)交换二叉链表树中所有左右子树
时间: 2024-12-17 08:32:15 浏览: 1
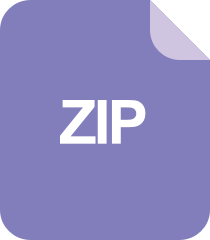
c语言编程题之树操作二叉树的最大深度.zip
好的,以下是利用C语言伪代码设计的四种算法:
**1. 计算二叉树节点个数(递归法)**
```c
int count_nodes_recursive(Node* root) {
if (root == NULL) return 0; // 空节点计数为0
return 1 + count_nodes_recursive(root->left) + count_nodes_recursive(root->right);
}
```
**2. 计算二叉树深度(递归法)**
```c
int max_depth(Node* root) {
if (root == NULL) return 0;
int left_depth = max_depth(root->left);
int right_depth = max_depth(root->right);
return (left_depth > right_depth ? left_depth : right_depth) + 1;
}
```
**3. 指定结点的层次数(非递归法,使用队列)**
```c
void level_number(Node* node, int level, int* result) {
if (node == NULL) return;
if (*result < level) *result = level;
queue_enqueue(node);
while (!queue_empty()) {
Node* current = queue_dequeue();
// 处理当前节点...
if (current->left != NULL) queue_enqueue(current->left);
if (current->right != NULL) queue_enqueue(current->right);
}
}
```
**4. 计算二叉链表树的叶结点数(递归法)**
```c
int leaf_count(Node* root) {
if (root == NULL) return 0;
if (root->left == NULL && root->right == NULL) return 1; // 叶节点直接返回1
return leaf_count(root->left) + leaf_count(root->right);
}
```
**5. 交换二叉链表树中所有左右子树(迭代法)**
```c
void swap_subtrees(Node* node) {
if (node == NULL || node->left == NULL || node->right == NULL) return;
Node* temp = node->left;
node->left = node->right;
node->right = temp;
// 递归处理左子树和右子树
swap_subtrees(node->left);
swap_subtrees(node->right);
}
```
阅读全文
相关推荐
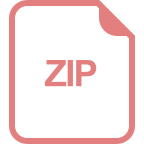
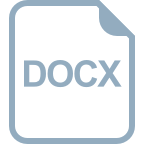
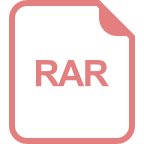
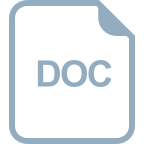













