上一题换一种方法,用《线性表的顺序存储结构——函数题》的知识
时间: 2024-09-23 14:17:04 浏览: 60
如果要用顺序存储(如数组)的方式来处理这一问题,我们可以创建一个新的数组,长度比原数组大两,用于存放插入后的有序序列。遍历原数组,将每个元素依次复制到新数组,然后在适当位置插入x和y。以下是使用这种方法的C语言代码:
```c
#include <stdio.h>
#define MAX_SIZE 100
// 用数组表示线性表
typedef struct {
int data[MAX_SIZE]; // 存储元素
int size; // 当前元素个数
} SequenceList;
// 函数原型,插入元素
void insert(SequenceList *list, int element) {
if (list->size == MAX_SIZE) {
printf("List is full.\n");
return;
}
list->data[list->size++] = element;
}
int main() {
int n, x, y;
SequenceList array;
// 初始化列表
scanf("%d", &n);
array.size = n;
// 读取元素并插入
for (int i = 0; i < n; ++i) {
scanf("%d", &array.data[i]);
}
scanf("%d %d", &x, &y);
// 插入元素x和y
insert(&array, x);
insert(&array, y);
// 输出结果
for (int i = 0; i <= array.size; ++i) { // 包含新插入的元素
printf("%d ", array.data[i]);
}
return 0;
}
```
在这个版本中,我们首先创建了一个顺序存储结构`SequenceList`,然后通过`insert`函数逐个插入元素。当列表满时,不再接受新的插入请求。
阅读全文
相关推荐
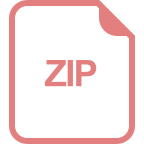
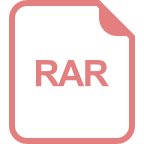
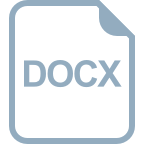
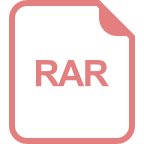
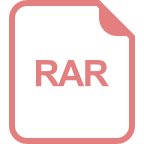
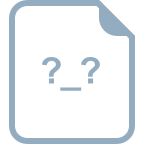
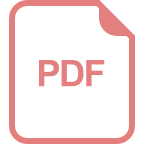
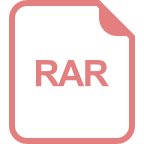
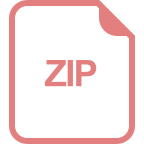
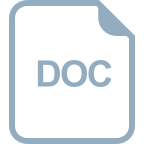
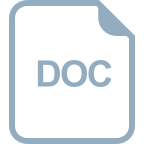
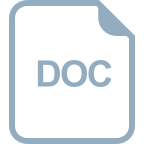
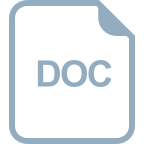
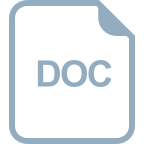
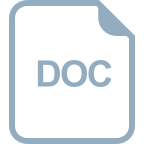
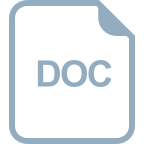
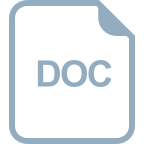

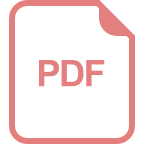