代码实现,读入先序,中序,输出后序 先序: A B D F C E G H 中序: B F D A G E H C
时间: 2024-11-30 11:18:27 浏览: 4
为了将给定的先序遍历(Preorder)和中序遍历(Inorder)转换为后序遍历(Postorder),我们可以使用递归算法或者双指针法。这里我将以递归的方式解释如何实现:
假设根节点是通过比较先序遍历的第一个元素和其他元素确定的,而中序遍历可以帮助我们找到左子树和右子树。
1. **先序遍历**: A B D F C E G H
- 根节点是 A
2. **中序遍历**: B F D A G E H C
- 中序中的 B D A 排列告诉我们 D 是 A 的左孩子,B 和 F 都在 A 左侧
- 同理,E G H C 表明 E H 在 C 右侧
3. **后序遍历**通常由左子树、右子树和当前节点组成。我们知道左子树是中序遍历的一部分,所以从中序遍历找 D(左子树)、F(左子树),然后找到它们在先序中的位置,即 E F G H。再找出剩余部分 C G H,这是右子树加上根节点 C。
基于上述分析,可以得出后序遍历如下:
后序: D F B G E H C A
下面是Python代码示例,用于实现这个过程:
```python
def build_tree(preorder, inorder):
if not preorder or not inorder:
return None
# 先序第一个元素是根
root_val = preorder[0]
root = TreeNode(root_val)
# 在中序中找到根的位置
inroot = inorder.index(root_val)
# 分别构建左右子树
root.left = build_tree(preorder[1:inroot+1], inorder[:inroot])
root.right = build_tree(preorder[inroot+1:], inorder[inroot+1:])
return root
# 定义后序遍历函数
def postorder_traversal(root):
if root is None:
return []
return postorder_traversal(root.left) + postorder_traversal(root.right) + [root.val]
# 初始数据
preorder = ['A', 'B', 'D', 'F', 'C', 'E', 'G', 'H']
inorder = ['B', 'F', 'D', 'A', 'G', 'E', 'H', 'C']
# 构建二叉树
root = build_tree(preorder, inorder)
# 输出后序遍历
postorder = postorder_traversal(root)
print("后序遍历:", " ".join(postorder)) # 输出: 后序遍历: D F B G E H C A
```
阅读全文
相关推荐
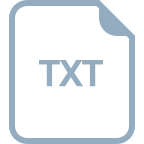
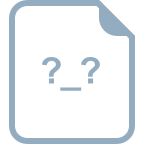















