c++ easyx 过一段时间再运行
时间: 2024-08-14 11:04:18 浏览: 28
EasyX是一个轻量级的C++游戏开发框架,主要用于Windows平台。它提供了一套简单易用的API来处理窗口、图形渲染、输入事件等基本游戏开发任务。如果你暂停了 EasyX 的程序并过一段时间后再运行,可能会遇到一些预期之外的问题:
1. 数据持久化:如果程序涉及到保存数据到文件,记得检查之前的数据是否已经被正确保存,并在重新加载。
2. 窗口状态:如果程序之前有打开窗口,再次运行前需要确认窗口是否关闭,否则可能会覆盖上一次的窗口。
3. 资源管理:比如图像、音频资源,如果没有正确的释放,可能会导致内存泄漏,所以需要确保资源在上次运行结束后已经正确卸载或释放。
4. 时间同步:游戏内的计时器、动画等可能依赖于上一次运行的状态,因此在重启时可能需要调整时间线。
5. 渲染设置:如果游戏中有自定义的图形设置,如分辨率、全屏模式等,需要确保这些设置与当前系统兼容。
相关问题
帮我用c++在easyx下写一段樱花树落叶的代码
好的,以下是一个使用EasyX图形库的C++代码,可以绘制一个樱花树落叶的动画:
```c++
#include <graphics.h>
#include <time.h>
const int WIDTH = 800; // 窗口宽度
const int HEIGHT = 600; // 窗口高度
const int TREE_X = 100; // 树的位置X坐标
const int TREE_Y = 500; // 树的位置Y坐标
const int TREE_WIDTH = 50; // 树的宽度
const int TREE_HEIGHT = 100; // 树的高度
const int LEAF_NUM = 50; // 叶子数量
// 叶子的结构体
struct Leaf
{
int x; // 叶子的位置X坐标
int y; // 叶子的位置Y坐标
int speed; // 叶子的下落速度
int angle; // 叶子的飘落角度
COLORREF color; // 叶子的颜色
};
// 初始化叶子
void initLeaves(Leaf leaves[])
{
srand((unsigned int)time(NULL));
for (int i = 0; i < LEAF_NUM; i++)
{
leaves[i].x = rand() % (TREE_WIDTH - 20) + TREE_X + 10;
leaves[i].y = rand() % (TREE_HEIGHT - 20) + TREE_Y - 70;
leaves[i].speed = rand() % 3 + 1;
leaves[i].angle = rand() % 60 + 240;
leaves[i].color = RGB(rand() % 256, rand() % 256, rand() % 256);
}
}
// 绘制樱花树
void drawTree()
{
setfillcolor(BROWN); // 设置树干颜色
solidrectangle(TREE_X, TREE_Y, TREE_X + TREE_WIDTH, TREE_Y + TREE_HEIGHT);
setfillcolor(PINK); // 设置花瓣颜色
fillcircle(TREE_X + TREE_WIDTH / 2, TREE_Y - 20, 30);
fillcircle(TREE_X + TREE_WIDTH / 2 - 20, TREE_Y - 50, 30);
fillcircle(TREE_X + TREE_WIDTH / 2 + 20, TREE_Y - 50, 30);
}
// 绘制叶子
void drawLeaf(Leaf leaf)
{
setfillcolor(leaf.color);
fillcircle(leaf.x, leaf.y, 5);
}
// 更新叶子的位置
void updateLeaf(Leaf& leaf)
{
leaf.x += leaf.speed * cos(leaf.angle * 3.14 / 180);
leaf.y += leaf.speed * sin(leaf.angle * 3.14 / 180);
// 叶子飘出窗口则重新生成
if (leaf.x < 0 || leaf.x > WIDTH || leaf.y > HEIGHT)
{
leaf.x = rand() % (TREE_WIDTH - 20) + TREE_X + 10;
leaf.y = rand() % (TREE_HEIGHT - 20) + TREE_Y - 70;
leaf.speed = rand() % 3 + 1;
leaf.angle = rand() % 60 + 240;
leaf.color = RGB(rand() % 256, rand() % 256, rand() % 256);
}
}
int main()
{
initgraph(WIDTH, HEIGHT); // 初始化窗口
Leaf leaves[LEAF_NUM];
initLeaves(leaves); // 初始化叶子
while (true)
{
drawTree(); // 绘制樱花树
for (int i = 0; i < LEAF_NUM; i++)
{
drawLeaf(leaves[i]); // 绘制叶子
updateLeaf(leaves[i]); // 更新叶子位置
}
Sleep(20); // 等待一段时间
cleardevice(); // 清空屏幕
}
closegraph(); // 关闭图形窗口
return 0;
}
```
运行代码将会显示一个樱花树落叶的动画效果。可以根据需要调整窗口大小、树的位置和大小、叶子数量和飘落速度等参数,以获得不同的效果。
黄金矿工 多文件 C++ easyx
这是一个简单的黄金矿工游戏的多文件 C++ 实现代码,使用了 EasyX 图形库。具体实现方法如下:
1. 创建一个 `main.cpp` 文件,用于包含主函数和游戏循环逻辑。
```c++
#include "Game.h"
#include <graphics.h>
int main()
{
initgraph(800, 600); // 初始化图形界面
Game game; // 创建游戏对象
game.start(); // 启动游戏循环
closegraph(); // 关闭图形界面
return 0;
}
```
2. 创建一个 `Game.h` 文件,用于定义游戏类。
```c++
#ifndef GAME_H
#define GAME_H
#include "Map.h"
#include "Player.h"
class Game
{
public:
Game();
void start();
private:
Map map;
Player player;
};
#endif // GAME_H
```
3. 创建一个 `Game.cpp` 文件,用于实现游戏类的方法。
```c++
#include "Game.h"
Game::Game()
{
map.init(); // 初始化地图
player.init(400, 500); // 初始化玩家
}
void Game::start()
{
while (!map.isGameOver()) // 游戏循环
{
map.draw(); // 绘制地图
player.draw(); // 绘制玩家
Sleep(10); // 延迟一段时间
cleardevice(); // 清空屏幕
}
}
```
4. 创建一个 `Map.h` 文件,用于定义地图类。
```c++
#ifndef MAP_H
#define MAP_H
#include <vector>
using namespace std;
class Map
{
public:
Map();
void init();
void draw();
bool isGameOver();
private:
vector<vector<int>> mapData;
int score;
};
#endif // MAP_H
```
5. 创建一个 `Map.cpp` 文件,用于实现地图类的方法。
```c++
#include "Map.h"
#include <graphics.h>
#include <time.h>
Map::Map()
{
score = 0; // 初始化分数
}
void Map::init()
{
srand(time(NULL)); // 设置随机数种子
for (int i = 0; i < 20; i++) // 初始化地图数据
{
vector<int> row;
for (int j = 0; j < 25; j++)
{
if (i == 0 || i == 19 || j == 0 || j == 24)
{
row.push_back(1); // 边界为石头
}
else
{
row.push_back(rand() % 3); // 其他位置为金子、石头或空地
}
}
mapData.push_back(row);
}
}
void Map::draw()
{
settextcolor(YELLOW);
settextstyle(16, 0, _T("宋体"));
outtextxy(10, 10, _T("得分:"));
TCHAR scoreStr[32];
_stprintf_s(scoreStr, _T("%d"), score);
outtextxy(70, 10, scoreStr); // 绘制分数
for (int i = 0; i < 20; i++)
{
for (int j = 0; j < 25; j++)
{
if (mapData[i][j] == 1)
{
setfillcolor(BROWN);
solidrectangle(j * 32, i * 32, j * 32 + 32, i * 32 + 32); // 绘制石头
}
else if (mapData[i][j] == 2)
{
setfillcolor(YELLOW);
solidcircle(j * 32 + 16, i * 32 + 16, 10); // 绘制金子
}
}
}
}
bool Map::isGameOver()
{
return false; // TODO: 实现游戏结束条件
}
```
6. 创建一个 `Player.h` 文件,用于定义玩家类。
```c++
#ifndef PLAYER_H
#define PLAYER_H
#include <graphics.h>
class Player
{
public:
Player();
void init(int x, int y);
void draw();
private:
int posX, posY;
};
#endif // PLAYER_H
```
7. 创建一个 `Player.cpp` 文件,用于实现玩家类的方法。
```c++
#include "Player.h"
Player::Player()
{
}
void Player::init(int x, int y)
{
posX = x;
posY = y;
}
void Player::draw()
{
setfillcolor(BLUE);
solidcircle(posX, posY, 10); // 绘制玩家
}
```
8. 最后,在项目中添加 EasyX 图形库的头文件和库文件,并编译运行即可。
注意:由于 EasyX 图形库只支持 Windows 系统,因此该代码只能在 Windows 系统下编译运行。
相关推荐
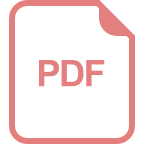
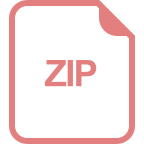
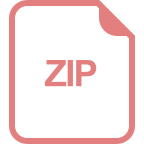












