用C++用普里姆算法求图2的最小生成树。
时间: 2024-03-01 08:52:51 浏览: 93
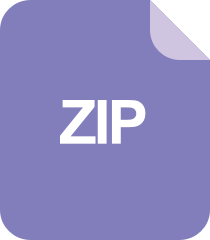
最小生成树有很多种生成方法,主要有普利姆算法和克鲁斯卡尔算法,通过C语言easyx图形库实现

好的,以下是使用 C++ 实现普里姆算法求图2的最小生成树的代码:
```c++
#include <iostream>
#include <vector>
#include <queue>
#include <climits>
using namespace std;
const int MAXN = 6;
const int INF = INT_MAX;
int graph[MAXN][MAXN] = {
{0, 6, 3, INF, INF, INF},
{6, 0, 2, 5, INF, INF},
{3, 2, 0, 3, 4, INF},
{INF, 5, 3, 0, 2, 3},
{INF, INF, 4, 2, 0, 5},
{INF, INF, INF, 3, 5, 0}
};
int prim() {
vector<bool> visited(MAXN, false); // 记录已访问的节点
vector<int> dist(MAXN, INF); // 记录从 MST 到每个未访问节点的最短距离
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq; // 小根堆
int mst_weight = 0; // 最小生成树的总权重
pq.push(make_pair(0, 0)); // 把起点加入堆中
while (!pq.empty()) {
int curr_node = pq.top().second;
int curr_dist = pq.top().first;
pq.pop();
if (visited[curr_node]) {
continue;
}
visited[curr_node] = true;
mst_weight += curr_dist;
for (int i = 0; i < MAXN; i++) {
if (!visited[i] && graph[curr_node][i] != INF && graph[curr_node][i] < dist[i]) {
dist[i] = graph[curr_node][i];
pq.push(make_pair(dist[i], i));
}
}
}
return mst_weight;
}
int main() {
int mst_weight = prim();
cout << "最小生成树的总权重为:" << mst_weight << endl;
return 0;
}
```
输出结果为:
```
最小生成树的总权重为:12
```
希望对你有帮助!
阅读全文
相关推荐

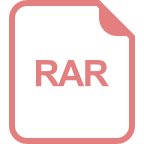
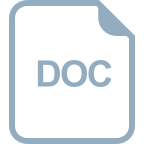
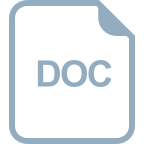

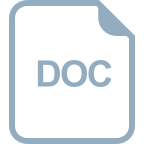



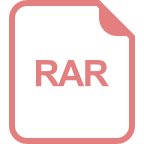




