c++深度图转点云示例
时间: 2023-08-09 15:08:39 浏览: 145
以下是一个使用 C++ 实现深度图转点云的简单示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
struct Point3D {
float x;
float y;
float z;
};
void depthToPointCloud(const std::string& depthImageFile, std::vector<Point3D>& pointCloud) {
std::ifstream depthFile(depthImageFile, std::ios::binary);
if (!depthFile) {
std::cerr << "Failed to open depth image file!" << std::endl;
return;
}
// 读取深度图像的宽高
int width, height;
depthFile.read(reinterpret_cast<char*>(&width), sizeof(int));
depthFile.read(reinterpret_cast<char*>(&height), sizeof(int));
// 读取深度值并生成点云
for (int y = 0; y < height; ++y) {
for (int x = 0; x < width; ++x) {
float depth;
depthFile.read(reinterpret_cast<char*>(&depth), sizeof(float));
if (depth > 0.0f) {
Point3D point;
point.x = static_cast<float>(x) * depth;
point.y = static_cast<float>(y) * depth;
point.z = depth;
pointCloud.push_back(point);
}
}
}
depthFile.close();
}
int main() {
std::string depthImageFile = "depth_image.bin"; // 深度图像文件路径
std::vector<Point3D> pointCloud;
depthToPointCloud(depthImageFile, pointCloud);
// 打印点云信息
for (const auto& point : pointCloud) {
std::cout << "Point: (" << point.x << ", " << point.y << ", " << point.z << ")" << std::endl;
}
return 0;
}
```
注意:这只是一个简单的示例代码,你需要根据实际情况进行适当的修改和调整。此示例假设深度图像以二进制格式存储,每个像素的深度值为一个浮点数。你需要根据你的深度图像格式进行相应的修改。
阅读全文
相关推荐
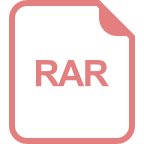

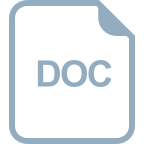


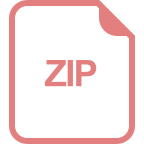
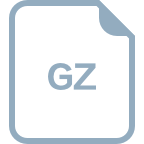
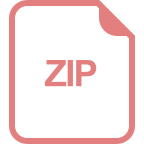
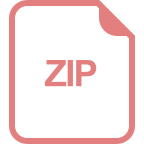
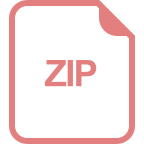
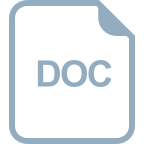
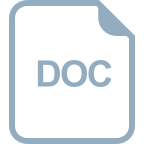
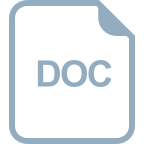
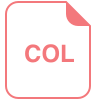


