能不能分别使用简单迭代法和快速迭代法写一个C++的程序,来求解方程“x-e^-x=0”精度为10^-6的根?
时间: 2024-10-07 09:05:54 浏览: 33
当然可以,这里我会给出使用C++分别用简单迭代法(固定点迭代)和快速迭代法(牛顿法)求解方程 \( x - e^{-x} = 0 \) 的示例,假设精度为 \( 10^{-6} \)。
**使用固定点迭代法 (简单迭代法)**:
```cpp
#include <iostream>
#include <cmath>
#include <vector>
double fixed_point_iterate(double guess, double tolerance = 1e-6) {
double function_value = std::exp(-guess) - guess;
while (std::abs(function_value) > tolerance) {
guess -= function_value;
function_value = std::exp(-guess) - guess;
}
return guess;
}
int main() {
double initial_guess = 0.0; // 初始猜测
double root = fixed_point_iterate(initial_guess);
std::cout << "Root found with simple iteration method: " << root << std::endl;
return 0;
}
```
**使用牛顿法 (快速迭代法)**:
```cpp
#include <iostream>
#include <cmath>
#include <vector>
// 定义函数f和它的导数f'
double f(double x) { return std::exp(- 1; }
double newton_iterate(double guess, double tolerance = 1e-6) {
double prev_root = guess;
while (true) {
double next_root = prev_root - f(prev_root) / df(prev_root);
if (std::abs(next_root - prev_root) <= tolerance) break;
prev_root = next_root;
}
return next_root;
}
int main() {
double initial_guess = 0.0; // 初始猜测
double root = newton_iterate(initial_guess);
std::cout << "Root found with Newton's method: " << root << std::endl;
return 0;
}
```
这两个程序都会尝试找到满足给定精度的方程根。在实际运行时,你可以根据需要调整初始猜测值和容忍度。记得检查代码是否正确实现了方程和相关的函数,并根据需要进行优化。
阅读全文
相关推荐
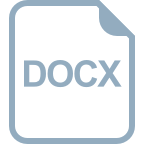
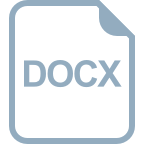
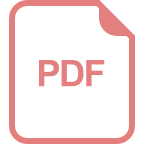









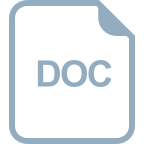




