Python解析串口GPS数据
时间: 2024-11-12 22:40:16 浏览: 28
在Python中解析串口GPS数据通常涉及到两个步骤:首先,你需要连接到串口设备并读取数据;其次,对接收到的数据进行解码和解析。
1. **连接串口**:
使用`pyserial`库可以很方便地处理串口通信。安装这个库后,你可以这样做:
```python
import serial
# 创建Serial对象
ser = serial.Serial(
port='COM1', # 替换为你设备的实际端口号
baudrate=9600, # 设置波特率,通常是9600、4800等
parity=serial.PARITY_NONE,
stopbits=serial.STOPBITS_ONE,
bytesize=serial.EIGHTBITS,
)
```
2. **读取数据**:
等待数据可用后,通过`ser.readline()`读取一行数据,它会返回包含换行符的新字符串。
3. **解析数据**:
GPS数据通常按照NMEA (National Marine Electronics Association) 格式发送,例如"$GPRMC"开始的行表示全球定位系统信息。你可以使用正则表达式或特定的库(如`gnss`或`u-blox-python`)来解析这些字段。比如,`RMC`消息有固定的结构,包括日期、时间、纬度、经度等信息。
```python
def parse_gprmc(line):
regex = r'^(?P<head>$GPRMC)\s+(?P<time>\d{2}:\d{2}.\d{2})\s+(?P<lat>[NS])(?P<lat_deg>\d{2})(\.(?P<lat_min>\d{2}))?\s+(?P<long>[EW])(?P<long_deg>\d{3})(\.(?P<long_min>\d{2}))?\s+'
match = re.match(regex, line.strip())
if match:
# 提取并处理各个字段
return {
'time': match.group('time'),
'latitude': f"{match.group('lat')}{match.group('lat_deg')}{'.' + match.group('lat_min') if match.group('lat_min') else ''}",
'longitude': f"{match.group('long')}{match.group('long_deg')}{'.' + match.group('long_min') if match.group('long_min') else ''}",
}
else:
return None
while True:
data = ser.readline().decode()
parsed_data = parse_gprmc(data)
if parsed_data:
print(parsed_data)
```
阅读全文
相关推荐
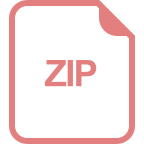
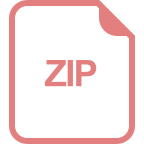
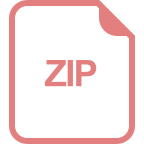








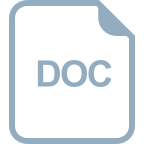






