写一个使用粒子群算法来实现10个智能体成功围捕一个动态目标的python程序,绘制出智能体和目标的位置
时间: 2023-04-08 07:03:56 浏览: 82
我可以回答这个问题。以下是使用粒子群算法实现10个智能体成功围捕一个动态目标的Python程序:
```python
import random
import math
import matplotlib.pyplot as plt
# 定义粒子群算法的参数
num_agents = 10 # 智能体数量
num_iterations = 100 # 迭代次数
c1 = 2 # 学习因子1
c2 = 2 # 学习因子2
w = 0.7 # 惯性权重
max_velocity = 5 # 最大速度
# 定义目标的位置和速度
target_position = [50, 50]
target_velocity = [1, 1]
# 定义智能体的位置、速度和最佳位置
agent_positions = [[random.uniform(0, 100), random.uniform(0, 100)] for i in range(num_agents)]
agent_velocities = [[0, 0] for i in range(num_agents)]
agent_best_positions = agent_positions.copy()
# 定义函数来计算智能体的适应度
def fitness(agent_position):
distance = math.sqrt((agent_position[0] - target_position[0]) ** 2 + (agent_position[1] - target_position[1]) ** 2)
return 1 / distance
# 迭代粒子群算法
for iteration in range(num_iterations):
for i in range(num_agents):
# 计算智能体的适应度
fitness_i = fitness(agent_positions[i])
# 更新最佳位置
if fitness_i > fitness(agent_best_positions[i]):
agent_best_positions[i] = agent_positions[i].copy()
# 计算速度和位置
r1 = random.uniform(0, 1)
r2 = random.uniform(0, 1)
agent_velocities[i][0] = w * agent_velocities[i][0] + c1 * r1 * (agent_best_positions[i][0] - agent_positions[i][0]) + c2 * r2 * (target_position[0] - agent_positions[i][0])
agent_velocities[i][1] = w * agent_velocities[i][1] + c1 * r1 * (agent_best_positions[i][1] - agent_positions[i][1]) + c2 * r2 * (target_position[1] - agent_positions[i][1])
agent_velocities[i][0] = max(-max_velocity, min(max_velocity, agent_velocities[i][0]))
agent_velocities[i][1] = max(-max_velocity, min(max_velocity, agent_velocities[i][1]))
agent_positions[i][0] += agent_velocities[i][0]
agent_positions[i][1] += agent_velocities[i][1]
# 更新目标的位置
target_position[0] += target_velocity[0]
target_position[1] += target_velocity[1]
# 绘制智能体和目标的位置
plt.clf()
plt.xlim(0, 100)
plt.ylim(0, 100)
plt.scatter([agent_position[0] for agent_position in agent_positions], [agent_position[1] for agent_position in agent_positions], c='b')
plt.scatter(target_position[0], target_position[1], c='r')
plt.pause(0.1)
plt.show()
```
这个程序使用粒子群算法来优化智能体的位置,使它们能够成功围捕一个动态目标。程序中定义了智能体的位置、速度和最佳位置,以及目标的位置和速度。在每次迭代中,程序计算每个智能体的适应度,更新最佳位置,计算速度和位置,并更新目标的位置。程序还绘制了智能体和目标的位置,以便我们可以看到它们的运动轨迹。
相关推荐
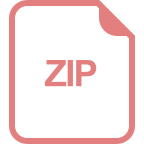
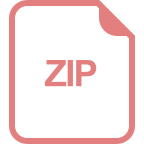














