一个函数的参数是回调函数,这个参数怎么写,例如:回调函数是void namesort(vector<string>& names,int idx = 0)
时间: 2024-03-05 11:53:59 浏览: 20
可以这样写:
```c++
void funcName(void (*callback)(vector<string>&, int), vector<string>& names, int idx = 0) {
callback(names, idx);
}
```
然后调用 `funcName` 函数时,将回调函数作为第一个参数传入即可。例如:
```c++
void myCallback(vector<string>& names, int idx) {
// 回调函数的具体实现
names[idx] = "new name";
}
vector<string> names = {"Alice", "Bob", "Charlie"};
funcName(myCallback, names, 1); // 调用时将 myCallback 作为回调函数传入
```
在上述代码中,`myCallback` 函数就是一个回调函数,用于在 `funcName` 函数内部被调用。`funcName` 函数的第一个参数就是回调函数,类型为 `void (*)(vector<string>&, int)`,表示一个参数为 `vector<string>&` 和 `int` 的无返回值函数指针。`names` 和 `idx` 则是回调函数的具体参数,由 `funcName` 函数传入。
相关问题
一个函数的参数是vector<string>类型,我该怎么传入实参
如果一个函数的参数是vector<string>类型,你可以按照以下方法来传入实参:
1. 直接传递一个vector<string>对象:
```c++
void func(vector<string> v) {
// 函数体
}
int main() {
vector<string> strVec = {"hello", "world"};
func(strVec); // 传递一个vector<string>对象
return 0;
}
```
2. 使用引用传递:
```c++
void func(vector<string>& v) {
// 函数体
}
int main() {
vector<string> strVec = {"hello", "world"};
func(strVec); // 传递一个vector<string>对象的引用
return 0;
}
```
使用引用传递可以避免在函数调用时拷贝vector<string>对象,减少了内存开销和时间消耗。同时,使用引用传递也可以在函数内部修改vector<string>对象。
3. 使用常量引用传递:
```c++
void func(const vector<string>& v) {
// 函数体
}
int main() {
vector<string> strVec = {"hello", "world"};
func(strVec); // 传递一个vector<string>对象的常量引用
return 0;
}
```
使用常量引用传递可以避免在函数调用时拷贝vector<string>对象,同时也可以防止函数内部修改vector<string>对象。
vector<vector<string>>作为函数参数时应该注意什么,并举例
当将 `vector<vector<string>>` 作为函数参数时,需要注意以下几点:
1. 确定函数参数类型为引用类型,以避免在函数调用过程中进行不必要的拷贝操作。
2. 确定函数参数类型为常量引用类型,以避免在函数内部修改函数参数。
下面是一个例子,假设我们需要实现一个函数来打印一个二维字符串数组:
```
void printStringArray(const vector<vector<string>>& arr) {
for (const auto& row : arr) {
for (const auto& col : row) {
cout << col << " ";
}
cout << endl;
}
}
```
在上面的函数中,我们将 `vector<vector<string>>` 定义为常量引用类型,并使用 `const auto&` 来遍历数组的每个元素。这样可以避免在函数内部修改函数参数,并且可以避免在函数调用过程中进行不必要的拷贝操作。
相关推荐
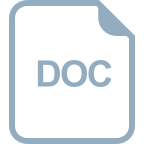













