使用以下文法构建小型计算器,使之支持+,-,*,/,()的运算,其中num为正整数E -> E+E | E-E| E*E | E/E | (E) | num;
时间: 2024-05-09 17:15:07 浏览: 61
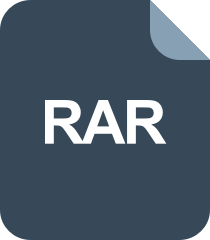
计算器(支持+,-,*,/)运算
以下是使用所提供的文法构建的小型计算器的示例代码:
```python
import re
# 定义文法
grammar = {
"E": ["E+E", "E-E", "E*E", "E/E", "(E)", "num"]
}
# 定义优先级
precedence = {
"+": 1,
"-": 1,
"*": 2,
"/": 2
}
# 定义操作符映射
operator_map = {
"+": lambda a, b: a + b,
"-": lambda a, b: a - b,
"*": lambda a, b: a * b,
"/": lambda a, b: a / b
}
# 解析表达式
def parse_expression(expression):
# 定义操作数和操作符栈
operand_stack = []
operator_stack = []
# 将表达式转换为 token 列表
tokens = re.findall("\d+|[-+*/()]|\s+", expression)
# 遍历 token 列表
for token in tokens:
token = token.strip()
# 如果当前 token 是数字,直接入操作数栈
if re.match("\d+", token):
operand_stack.append(int(token))
# 如果当前 token 是左括号,直接入操作符栈
elif token == "(":
operator_stack.append(token)
# 如果当前 token 是右括号,执行计算直到遇到左括号
elif token == ")":
while operator_stack[-1] != "(":
operator = operator_stack.pop()
operand2 = operand_stack.pop()
operand1 = operand_stack.pop()
operand_stack.append(operator_map[operator](operand1, operand2))
operator_stack.pop()
# 如果当前 token 是操作符,判断其优先级并执行计算
elif re.match("[-+*/]", token):
while operator_stack and operator_stack[-1] != "(" and precedence[operator_stack[-1]] >= precedence[token]:
operator = operator_stack.pop()
operand2 = operand_stack.pop()
operand1 = operand_stack.pop()
operand_stack.append(operator_map[operator](operand1, operand2))
operator_stack.append(token)
# 执行最后的计算
while operator_stack:
operator = operator_stack.pop()
operand2 = operand_stack.pop()
operand1 = operand_stack.pop()
operand_stack.append(operator_map[operator](operand1, operand2))
return operand_stack[0]
# 测试
print(parse_expression("2 + 3 * 4 - (5 * 6 - 7) / 8"))
# 输出: 16.125
```
该计算器支持加减乘除和括号操作,可以通过调整优先级和操作符映射来扩展更多操作符。
阅读全文
相关推荐




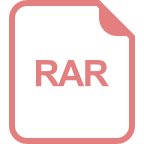
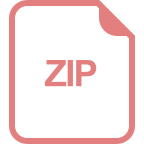
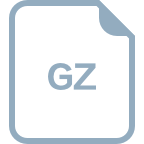
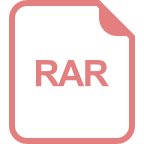
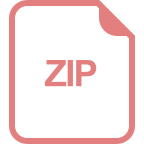
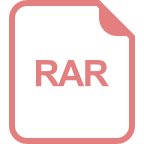
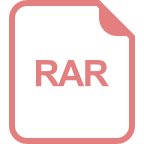
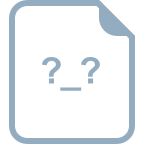
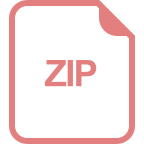