c语言将运算表达式转化为后缀式表达式的代码
时间: 2024-06-11 12:09:59 浏览: 135
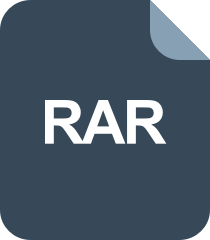
算数表达式转后缀表达式C++
以下是将运算表达式转化为后缀式表达式的C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_SIZE 100
typedef struct {
char data[MAX_SIZE];
int top;
} Stack;
void init_stack(Stack *s) {
s->top = -1;
}
void push(Stack *s, char c) {
if (s->top == MAX_SIZE - 1) {
printf("Stack overflow\n");
exit(1);
}
s->top++;
s->data[s->top] = c;
}
char pop(Stack *s) {
if (s->top == -1) {
printf("Stack underflow\n");
exit(1);
}
char c = s->data[s->top];
s->top--;
return c;
}
int is_operator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/');
}
int precedence(char c) {
if (c == '+' || c == '-') {
return 1;
} else if (c == '*' || c == '/') {
return 2;
} else {
return 0;
}
}
void infix_to_postfix(char infix[], char postfix[]) {
Stack s;
init_stack(&s);
int i = 0, j = 0;
while (infix[i] != '\0') {
char c = infix[i];
if (isdigit(c)) {
postfix[j] = c;
j++;
} else if (is_operator(c)) {
while (s.top != -1 && is_operator(s.data[s.top]) && precedence(s.data[s.top]) >= precedence(c)) {
postfix[j] = pop(&s);
j++;
}
push(&s, c);
} else if (c == '(') {
push(&s, c);
} else if (c == ')') {
while (s.top != -1 && s.data[s.top] != '(') {
postfix[j] = pop(&s);
j++;
}
if (s.top == -1) {
printf("Unmatched parenthesis\n");
exit(1);
}
pop(&s);
} else {
printf("Invalid character\n");
exit(1);
}
i++;
}
while (s.top != -1) {
if (s.data[s.top] == '(') {
printf("Unmatched parenthesis\n");
exit(1);
}
postfix[j] = pop(&s);
j++;
}
postfix[j] = '\0';
}
int main() {
char infix[MAX_SIZE], postfix[MAX_SIZE];
printf("Enter infix expression: ");
fgets(infix, MAX_SIZE, stdin);
infix_to_postfix(infix, postfix);
printf("Postfix expression: %s\n", postfix);
return 0;
}
```
使用方法:
1. 编译代码:
```
gcc -o postfix postfix.c
```
2. 运行程序:
```
./postfix
```
3. 输入中缀表达式,按回车键:
```
Enter infix expression: (3+4)*5-6/2
```
4. 程序输出后缀表达式:
```
Postfix expression: 34+5*62/-
```
阅读全文
相关推荐
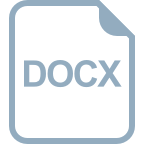

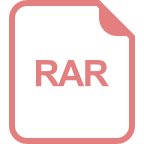
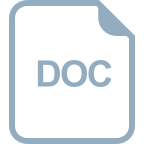
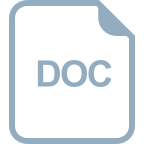
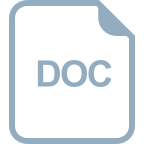
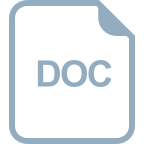
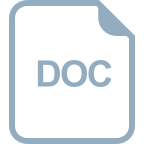







