滚动在线RRT算法matlab
时间: 2023-09-06 18:07:10 浏览: 102
以下是一个简单的Matlab实现滚动在线RRT算法的示例代码:
```matlab
function [path,tree] = rolling_rrt(start,goal,obstacles,max_iter,delta,q,step_size)
% start: 起点,格式为[x,y]
% goal: 终点,格式为[x,y]
% obstacles: 障碍物,格式为x-y-z三维矩阵,其中x、y为障碍物的位置坐标,z为障碍物半径
% max_iter: 迭代次数
% delta: 随机采样点生成概率
% q: 路径连接概率
% step_size: 步长
% path: 找到的路径,格式为[x1,y1;x2,y2;...;xn,yn]
% tree: RRT树,格式为x-y-z三维矩阵,其中x、y为节点的位置坐标,z为节点的父节点序号
% 初始化RRT树
tree = [start,0];
n_nodes = 1;
for i = 1:max_iter
% 随机采样
if rand < delta
sample = goal;
else
sample = [rand*10,rand*10];
end
% 查找最近节点
min_dist = Inf;
min_node = [];
for j = 1:n_nodes
dist = norm(tree(j,1:2)-sample);
if dist < min_dist
min_dist = dist;
min_node = j;
end
end
% 扩展节点
new_node = tree(min_node,1:2) + step_size*(sample-tree(min_node,1:2))/min_dist;
if ~check_collision(new_node,obstacles)
% 查找最近邻节点
min_dist = Inf;
min_index = [];
for j = 1:n_nodes
if j == min_node
continue;
end
dist = norm(tree(j,1:2)-new_node);
if dist < min_dist
min_dist = dist;
min_index = j;
end
end
% 连接路径
if rand < q
if ~check_collision(new_node,obstacles)
tree = [tree;new_node,min_node];
n_nodes = n_nodes + 1;
if min_index ~= []
tree(n_nodes,3) = min_index;
end
if norm(new_node-goal) < step_size
break;
end
end
end
end
end
% 从终点回溯路径
path = goal;
node = n_nodes;
while node ~= 1
path = [tree(node,1:2);path];
node = tree(node,3);
end
path = [start;path];
end
function collision = check_collision(point,obstacles)
% 检查是否碰撞
collision = false;
for i = 1:size(obstacles,1)
if norm(point-obstacles(i,1:2)) < obstacles(i,3)
collision = true;
break;
end
end
end
```
这是一个简单的示例,仅用于演示RRT算法的基本思想。实际使用时需要根据具体问题进行修改和优化。
阅读全文
相关推荐
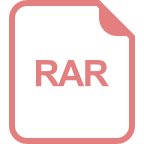
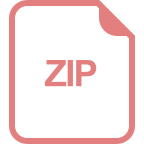
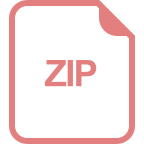
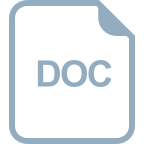
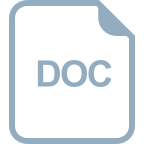
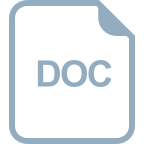









