public class Test { public int aMethod() { static int i = 0; i++; return i; } public static void main (String args[]) { Test test = new Test(); test.aMethod(); int j = test.aMethod(); System.out.println(j); } }
时间: 2023-11-22 13:56:37 浏览: 385
这段代码有语法错误,无法通过编译。静态变量不能在方法内部声明。正确的写法应该是将静态变量 i 声明在方法外部,如下所示:
```
public class Test {
static int i = 0;
public int aMethod() {
i++;
return i;
}
public static void main(String[] args) {
Test test = new Test();
test.aMethod();
int j = test.aMethod();
System.out.println(j);
}
}
```
此时输出结果为 2,因为 i 的值在两次调用 aMethod 方法时分别增加了 1。
相关问题
如下代码,输出结果是什么? public class Test { public int aMethod() { static int i = 0; i++; return i; } public static void main (String args[]) { Test test = new Test(); test.aMethod(); int j = test.aMethod(); System.out.println(j); } }
这段代码是有语法错误的,因为在方法 aMethod() 中定义了一个静态变量 i,但是静态变量不能在方法中定义,只能在类中定义。
如果将静态变量 i 定义在类中,则输出的结果是 2。因为第一次调用 aMethod() 方法时,i 的值从 0 变为 1,并将 1 返回;第二次调用 aMethod() 方法时,i 的值从 1 变为 2,并将 2 返回。最后输出的 j 的值是 2。以下是修正后的代码:
```
public class Test {
static int i = 0;
public int aMethod() {
i++;
return i;
}
public static void main (String args[]) {
Test test = new Test();
test.aMethod();
int j = test.aMethod();
System.out.println(j);
}
}
```
以下程序运行结果正确的是:【 】 class Base { public void method() { System.out.println("不带参Base"); } } public class Sub extends Base { public void method() { System.out.println("不带参Sub"); } public int method(int a) { System.out.println("带参Sub"); return 0; } public static void main(String[] args) { Sub test = new Sub(); test.method(); test.method(0); } }
以下程序运行结果正确的是:【 √ 】运行结果为:
不带参Sub
带参Sub
这是因为Sub类继承了Base类,并覆盖了Base类中的method方法,当调用Sub类的method方法时,会优先调用Sub类的方法。而Sub类中还定义了一个带有int参数的method方法,当调用该方法时,会根据参数类型的不同,调用相应的方法。因此,在main方法中,先调用了不带参的method方法,输出"不带参Sub",然后调用了带参的method方法,输出"带参Sub"。
阅读全文
相关推荐
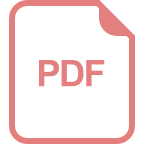
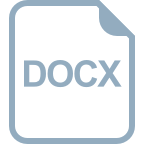
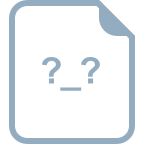














