ctx === null || ctx === void 0 ? void 0 : ctx.fillStyle = 'green';
时间: 2023-08-25 20:14:39 浏览: 40
这是一个JavaScript语句,用于设置Canvas 2D上下文的填充颜色。它使用了可选链运算符(?.)来避免在Canvas 2D上下文对象不存在的情况下引发错误。如果ctx对象为null或undefined,则该语句将不执行任何操作,而是直接返回。
在Canvas 2D上下文中,fillStyle属性用于设置填充颜色。它可以接受以下几种值:
- 颜色名称:例如'red'、'green'等。
- RGB值:例如'rgb(255,0,0)'、'rgb(0,255,0)'等。
- RGBA值:例如'rgba(255,0,0,0.5)'、'rgba(0,255,0,0.5)'等。
- 十六进制值:例如'#FF0000'、'#00FF00'等。
- 渐变对象:例如线性渐变、径向渐变等。
- 图案对象:例如图像、Canvas元素等。
在这个例子中,我们将填充颜色设置为绿色('green'),这意味着在绘制时,我们将使用绿色填充任何形状(例如矩形、圆形、路径等)。由于该语句的位置在fillRect()方法之后,因此我们将在填充黑色矩形的上方使用绿色填充。
相关问题
ctx.fillStyle="red" 无效
您好!对于您提到的问题,可能有几种原因导致 `ctx.fillStyle="red"` 无效。下面是一些常见的原因和解决方法:
1. 确保您正在使用正确的上下文对象:首先,确保您正确地获取了绘图上下文对象。例如,如果您使用的是 Canvas 元素,您需要使用 `getContext` 方法来获取 2D 上下文对象,例如:`var ctx = canvas.getContext("2d");`。然后,您可以在此对象上设置 `fillStyle`。
2. 确保您正在正确设置颜色值:在设置 `fillStyle` 时,确保您正在使用有效的颜色值。例如,可以使用 CSS 颜色名称(如 "red")或十六进制颜色代码(如 "#FF0000")。
3. 确保您在绘制之前设置了 `fillStyle`:请确保在执行绘制操作(如 `fillRect`)之前设置了 `fillStyle`。否则,颜色设置可能不会生效。
以下是一个示例代码片段,展示了如何正确设置 `fillStyle` 并绘制一个红色矩形:
```javascript
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
ctx.fillStyle = "red"; // 设置颜色为红色
ctx.fillRect(10, 10, 100, 100); // 绘制红色矩形
```
希望这些信息对您有所帮助!如果您有任何其他问题,请随时提问。
class Player { constructor(x, y, radius, color, vector) { this.x = x; this.y = y; this.radius = radius; this.color = color; this.vector = vector; this.life = 3;//玩家生命值 } draw(ctx) { ctx.save(); ctx.beginPath(); ctx.fillStyle = this.color; ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false); ctx.fill(); ctx.closePath(); ctx.restore(); } resize(x, y) { this.x = x; this.y = y; } } class Enemy { constructor(x, y, radius, color, vector) { this.x = x; this.y = y; this.radius = radius; this.color = color; this.vector = vector; } draw(ctx) { ctx.save(); ctx.beginPath(); ctx.fillStyle = this.color; ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false); ctx.fill(); ctx.closePath(); ctx.restore(); } update() { this.x += this.vector.x; this.y += this.vector.y; } } 要为玩家添加生命值。判断敌人与玩家是否撞击,是则玩家的生命值减1当玩家生命值减到0时,游戏结束。
可以在 `Player` 类中添加一个 `life` 属性来表示玩家的生命值,并将其初始化为 3。然后在 `Enemy` 类中添加一个 `checkCollision()` 方法来检测敌人与玩家是否撞击,如果撞击,则将玩家的生命值减 1。在 `Player` 类中添加一个 `checkGameOver()` 方法来检测玩家生命值是否减为 0,如果减为 0,则游戏结束。
下面是修改后的代码:
```
class Player {
constructor(x, y, radius, color, vector) {
this.x = x;
this.y = y;
this.radius = radius;
this.color = color;
this.vector = vector;
this.life = 3; // 玩家生命值
}
draw(ctx) {
ctx.save();
ctx.beginPath();
ctx.fillStyle = this.color;
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false);
ctx.fill();
ctx.closePath();
ctx.restore();
}
resize(x, y) {
this.x = x;
this.y = y;
}
checkGameOver() {
if (this.life === 0) {
// 游戏结束
console.log('Game over');
}
}
}
class Enemy {
constructor(x, y, radius, color, vector) {
this.x = x;
this.y = y;
this.radius = radius;
this.color = color;
this.vector = vector;
}
draw(ctx) {
ctx.save();
ctx.beginPath();
ctx.fillStyle = this.color;
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false);
ctx.fill();
ctx.closePath();
ctx.restore();
}
update() {
this.x += this.vector.x;
this.y += this.vector.y;
}
checkCollision(player) {
const distance = Math.sqrt((player.x - this.x) ** 2 + (player.y - this.y) ** 2);
if (distance < player.radius + this.radius) {
// 玩家与敌人撞击,玩家生命值减1
player.life--;
console.log('Life:', player.life);
}
}
}
```
相关推荐
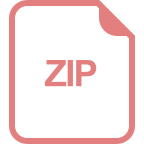
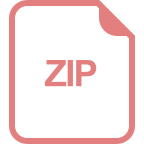













