中缀表达式转后缀表达式的c语言代码加解释
时间: 2023-10-16 16:11:57 浏览: 90
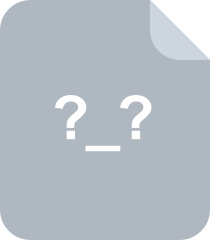
中缀转后缀_C语言代码
下面是中缀表达式转后缀表达式的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX 100
char stack[MAX];
int top = -1;
int is_operator(char ch) {
return (ch == '+' || ch == '-' || ch == '*' || ch == '/');
}
int precedence(char ch) {
if (ch == '+' || ch == '-')
return 1;
else if (ch == '*' || ch == '/')
return 2;
else
return 0;
}
void push(char ch) {
if (top == MAX - 1) {
printf("Stack Overflow\n");
exit(1);
}
stack[++top] = ch;
}
char pop() {
if (top == -1) {
printf("Stack Underflow\n");
exit(1);
}
return stack[top--];
}
void infix_to_postfix(char infix[], char postfix[]) {
int i, j;
char ch;
for (i = 0, j = 0; infix[i] != '\0'; i++) {
if (infix[i] == '(')
push(infix[i]);
else if (infix[i] == ')') {
while (stack[top] != '(')
postfix[j++] = pop();
pop(); // remove '('
}
else if (!is_operator(infix[i])) {
postfix[j++] = infix[i];
}
else {
while (precedence(infix[i]) <= precedence(stack[top]))
postfix[j++] = pop();
push(infix[i]);
}
}
while (top != -1)
postfix[j++] = pop();
postfix[j] = '\0';
}
int main() {
char infix[MAX], postfix[MAX];
printf("Enter infix expression: ");
scanf("%s", infix);
infix_to_postfix(infix, postfix);
printf("Postfix expression: %s\n", postfix);
return 0;
}
```
代码的基本思路是利用栈,从左到右遍历中缀表达式,遇到操作数则直接输出到后缀表达式中,遇到左括号则压入栈中,遇到右括号则弹出栈中的元素,直到遇到左括号为止,并将左括号弹出,遇到操作符时弹出栈顶元素,直到栈顶元素的优先级小于当前操作符的优先级,然后将当前操作符压入栈中。
对于每个字符,都要进行判断,判断是不是操作符,是不是左括号或右括号,或者是操作数。其中的is_operator函数用于判断一个字符是否是操作符,precedence函数用于返回一个操作符的优先级。
注:此代码只能处理单个数字,不能处理多位数字,如需处理多位数字,需要对代码进行修改。
阅读全文
相关推荐




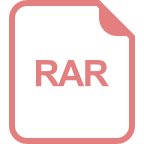









