中缀表达式转后缀表达式c语言代码
时间: 2023-07-31 07:13:42 浏览: 124
好的,以下是中缀表达式转后缀表达式的C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_LEN 100 // 定义最大表达式长度
typedef struct {
char data[MAX_LEN];
int top;
} Stack;
// 初始化栈
void InitStack(Stack *s) {
s->top = -1;
}
// 判断栈是否为空
int IsEmpty(Stack *s) {
return s->top == -1;
}
// 判断栈是否已满
int IsFull(Stack *s) {
return s->top == MAX_LEN - 1;
}
// 入栈操作
void Push(Stack *s, char ch) {
if (IsFull(s)) {
printf("Error: stack is full!\n");
exit(1);
}
s->top++;
s->data[s->top] = ch;
}
// 出栈操作
char Pop(Stack *s) {
if (IsEmpty(s)) {
printf("Error: stack is empty!\n");
exit(1);
}
char ch = s->data[s->top];
s->top--;
return ch;
}
// 获取栈顶元素
char GetTop(Stack *s) {
if (IsEmpty(s)) {
printf("Error: stack is empty!\n");
exit(1);
}
return s->data[s->top];
}
// 获取运算符优先级
int GetPriority(char ch) {
if (ch == '+' || ch == '-') {
return 1;
} else if (ch == '*' || ch == '/') {
return 2;
} else {
return 0;
}
}
void InfixToSuffix(char *infix, char *suffix) {
Stack s;
InitStack(&s);
int len = strlen(infix);
int j = 0;
for (int i = 0; i < len; i++) {
char ch = infix[i];
if (isdigit(ch)) { // 如果是数字,直接添加到后缀表达式中
suffix[j++] = ch;
} else if (ch == '(') { // 如果是左括号,入栈
Push(&s, ch);
} else if (ch == ')') { // 如果是右括号,依次弹出栈顶元素直到遇到左括号
char top_ch = GetTop(&s);
while (top_ch != '(') {
suffix[j++] = top_ch;
Pop(&s);
top_ch = GetTop(&s);
}
Pop(&s); // 弹出左括号
} else if (ch == '+' || ch == '-' || ch == '*' || ch == '/') { // 如果是运算符
while (!IsEmpty(&s) && GetPriority(ch) <= GetPriority(GetTop(&s))) {
// 当前运算符优先级小于等于栈顶运算符优先级,弹出栈顶元素添加到后缀表达式中
suffix[j++] = Pop(&s);
}
Push(&s, ch); // 当前运算符入栈
} else {
printf("Error: invalid character!\n");
exit(1);
}
}
// 将栈中剩余元素依次弹出添加到后缀表达式中
while (!IsEmpty(&s)) {
suffix[j++] = Pop(&s);
}
suffix[j] = '\0'; // 添加字符串结束符
}
int main() {
char infix[MAX_LEN], suffix[MAX_LEN];
printf("请输入中缀表达式:");
scanf("%s", infix);
InfixToSuffix(infix, suffix);
printf("后缀表达式为:%s\n", suffix);
return 0;
}
```
以上是中缀表达式转后缀表达式的C语言代码实现,希望能对您有所帮助。
阅读全文
相关推荐
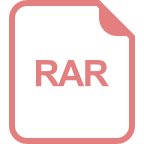






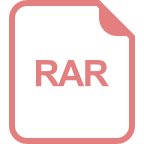






