pandas 追加数据
时间: 2023-06-29 13:19:11 浏览: 88
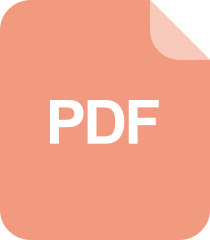
Python pandas如何向excel添加数据
在 pandas 中,可以使用 `DataFrame` 的 `append()` 方法将新的数据追加到现有的数据中。例如,假设有一个名为 `df` 的 `DataFrame`,需要将一个名为 `new_data` 的数据追加到其中:
``` python
df = df.append(new_data, ignore_index=True)
```
其中,`ignore_index=True` 参数表示忽略现有数据索引,按顺序添加新的数据。你也可以使用 `concat()` 方法来实现相同的效果:
``` python
df = pd.concat([df, new_data], ignore_index=True)
```
需要注意的是,追加数据的频繁操作会导致性能问题,应该尽可能避免。如果需要处理大量数据,可以考虑使用 `pandas` 的其他高效方法,例如 `pd.read_csv()`、`pd.read_sql()` 等。
阅读全文
相关推荐












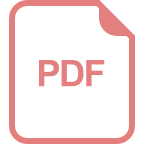
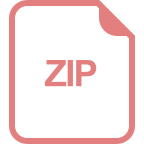
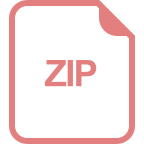