矩阵的加法运算C++
时间: 2024-11-02 13:07:10 浏览: 9
矩阵的加法运算是线性的,它涉及到两个同样大小的矩阵元素对应位置的相加。在C++中,如果你想要处理二维数组(矩阵)的加法,你可以创建一个函数,接受两个矩阵作为输入,并返回一个新的矩阵,其中每个元素是输入矩阵对应位置元素之和。下面是一个简单的示例:
```cpp
#include <iostream>
using namespace std;
// 定义矩阵类,包含维度和数据成员
class Matrix {
private:
int rows;
int cols;
double data[100][100]; // 可能需要调整大小以适应实际需求
public:
// 构造函数等...
Matrix(int r, int c) : rows(r), cols(c) {}
// 加法运算
Matrix operator+(const Matrix &other) {
if (rows != other.rows || cols != other.cols) {
throw runtime_error("Matrices must have the same dimensions for addition");
}
Matrix result(rows, cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
result.data[i][j] = data[i][j] + other.data[i][j];
}
}
return result;
}
// 输出矩阵
void display() const {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
cout << data[i][j] << " ";
}
cout << endl;
}
}
};
int main() {
Matrix m1(2, 2), m2(2, 2);
// 初始化矩阵...
m1.display(); // 显示m1
m2.display(); // 显示m2
Matrix sum = m1 + m2;
cout << "Sum of matrices:" << endl;
sum.display();
return 0;
}
```
在这个例子中,我们定义了一个Matrix类,通过重载`+`操作符实现了矩阵的加法。注意,这个实现假设了矩阵的行数和列数是一致的。如果尝试添加不同尺寸的矩阵,会抛出异常。
阅读全文
相关推荐
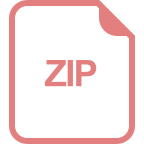
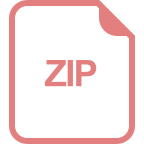
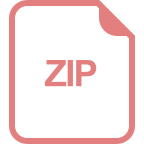
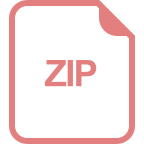

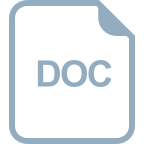
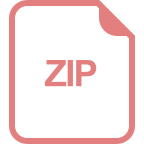
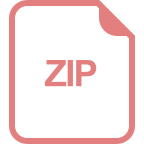
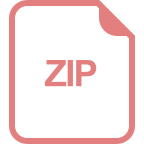
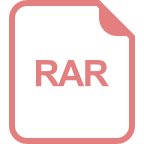
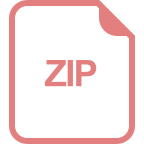
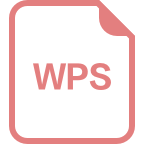
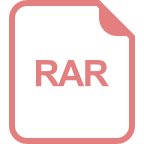
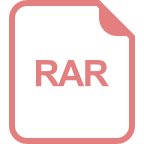
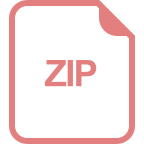



