color = cv2.imread(color_dir).astype(np.float32) / 255.0 depth = cv2.imread(depth_dir, cv2.IMREAD_UNCHANGED).astype(np.float32) / 1000.0
时间: 2024-04-18 08:30:12 浏览: 151
这段代码使用OpenCV库加载图像文件,并将图像转换为浮点型数组。
首先,`cv2.imread(color_dir)`加载了名为`color_dir`的彩色图像文件,并将其存储在变量`color`中。这个函数返回一个NumPy数组,其中包含图像的像素值。
然后,`.astype(np.float32)`将图像数组的数据类型转换为浮点型(32位浮点数)。这是因为后续的计算通常需要在浮点数域上进行。
接下来,除以255.0将像素值范围从[0, 255]归一化到[0, 1]。这样做是为了确保图像的像素值处于0到1之间,方便后续的处理和计算。
类似地,`cv2.imread(depth_dir, cv2.IMREAD_UNCHANGED)`加载了名为`depth_dir`的深度图像文件,并将其存储在变量`depth`中。`cv2.IMREAD_UNCHANGED`参数告诉OpenCV保持深度图像的原始通道数和数据类型。
最后,`.astype(np.float32) / 1000.0`将深度图像数组的数据类型转换为浮点型,并将深度值范围从毫米转换为米。这样做是为了确保深度图像的值处于0到1之间,并采用与彩色图像类似的数据范围。
通过这些转换,你可以在后续的图像处理或计算过程中使用浮点型的彩色图像和深度图像。
相关问题
def extract_building_shadow(image, dsm, ground_spacing, radius): shadow_mask = np.zeros_like(image, dtype=np.bool) for i in range(0, image.shape[0], ground_spacing): for j in range(0, image.shape[1], ground_spacing): if not np.any(shadow_mask[i, j]): center = (i, j) ground_height = dsm[i, j] for x, y in spiral_search(center, radius, ground_spacing): if x < 0 or x >= image.shape[0] or y < 0 or y >= image.shape[1]: continue if np.any(shadow_mask[x, y:]): continue height = dsm[x, y] if height > ground_height: shadow_mask[x, y] = True elif height == ground_height: if np.linalg.norm(np.array([x, y]) - np.array(center)) < \ np.linalg.norm(np.array([i, j]) - np.array(center)): shadow_mask[x, y] = True return shadow_mask image = cv2.imread('C:\yingxiang\DJI_20230322140516_0026_V.JPG') dsm_path = 'C:/sanwei/jianmo/Productions/Production_2/Production_2_DSM_part_2_2.tif' dsm_dataset = gdal.Open(dsm_path) output_path = 'C:\yingxiang\shadow_mask.png'这段代码怎么改可以将掩膜结果保存
您可以在函数返回结果时添加以下代码将掩膜结果保存为 PNG 文件:
```
cv2.imwrite(output_path, shadow_mask.astype(np.uint8) * 255)
```
这将把 `shadow_mask` 数组中的布尔值转换为 0 和 1 的整数,并将其乘以 255 然后转换为无符号 8 位整数,最后使用 OpenCV 的 `imwrite()` 函数将其保存为 PNG 文件。请确保 `output_path` 变量包含正确的文件路径和文件名。
from skimage.segmentation import slic, mark_boundaries import torchvision.transforms as transforms import numpy as np from PIL import Image import matplotlib.pyplot as plt # 加载图像 image = Image.open('3.jpg') # 转换为 PyTorch 张量 transform = transforms.ToTensor() img_tensor = transform(image).unsqueeze(0) # 将 PyTorch 张量转换为 Numpy 数组 img_np = img_tensor.numpy().transpose(0, 2, 3, 1)[0] # 使用 SLIC 算法生成超像素标记图 segments = slic(img_np, n_segments=60, compactness=10) # 可视化超像素索引映射 plt.imshow(segments, cmap='gray') plt.show() # 将超像素索引映射可视化 segment_img = mark_boundaries(img_np, segments) # 将 Numpy 数组转换为 PIL 图像 segment_img = Image.fromarray((segment_img * 255).astype(np.uint8)) # 保存超像素索引映射可视化 segment_img.save('segment_map.jpg') 将上述代码中引入超像素池化代码:import cv2 import numpy as np # 读取图像 img = cv2.imread('3.jpg') # 定义超像素分割器 num_segments = 60 # 超像素数目 slic = cv2.ximgproc.createSuperpixelSLIC(img, cv2.ximgproc.SLICO, num_segments) # 进行超像素分割 slic.iterate(10) # 获取超像素标签和数量 labels = slic.getLabels() num_label = slic.getNumberOfSuperpixels() # 对每个超像素进行池化操作,这里使用平均值池化 pooled = [] for i in range(num_label): mask = labels == i region = img[mask] pooled.append(region.mean(axis=0)) # 将池化后的特征图可视化 pooled = np.array(pooled, dtype=np.uint8) pooled_features = pooled.reshape(-1) pooled_img = cv2.resize(pooled_features, (img.shape[1], img.shape[0]), interpolation=cv2.INTER_NEAREST) print(pooled_img.shape) cv2.imshow('Pooled Image', pooled_img) cv2.waitKey(0),并显示超像素池化后的特征图
好的,这个问题是要求你在给定的代码中添加超像素池化的实现,并显示超像素池化后的特征图。以下是添加超像素池化的代码和显示超像素池化后的特征图的代码:
```
import cv2
import numpy as np
# 读取图像
img = cv2.imread('3.jpg')
# 定义超像素分割器
num_segments = 60 # 超像素数目
slic = cv2.ximgproc.createSuperpixelSLIC(img, cv2.ximgproc.SLICO, num_segments)
# 进行超像素分割
slic.iterate(10)
# 获取超像素标签和数量
labels = slic.getLabels()
num_label = slic.getNumberOfSuperpixels()
# 对每个超像素进行池化操作,这里使用平均值池化
pooled = []
for i in range(num_label):
mask = labels == i
region = img[mask]
pooled.append(region.mean(axis=0))
# 将池化后的特征图可视化
pooled = np.array(pooled, dtype=np.uint8)
pooled_features = pooled.reshape(-1)
pooled_img = cv2.resize(pooled_features, (img.shape[1], img.shape[0]), interpolation=cv2.INTER_NEAREST)
# 显示超像素池化后的特征图
cv2.imshow('Pooled Image', pooled_img.reshape(img.shape))
cv2.waitKey(0)
```
注意,这里使用的是 OpenCV 库中的超像素分割器,并且超像素池化使用的是平均值池化。最后,使用 OpenCV 库中的 imshow() 函数和 waitKey() 函数来显示超像素池化后的特征图。
阅读全文
相关推荐
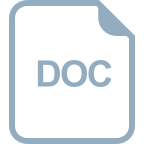
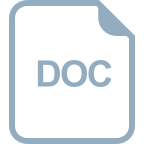
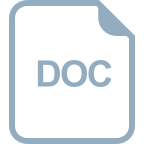














