桌上有一空盒子,允许存放一个球,老师可以往盒子中放红球,也可放绿球,小红只准取红球,小明只准取绿球,规定当盒子空时,一次只能放一个球供他们取用,请用 PV原语实现老师、小红和小明三人并发进程的同步。
时间: 2024-10-10 17:02:43 浏览: 22
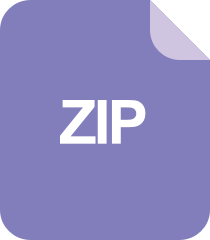
红黄蓝绿四色球数据-1745张-含voc(xml)+yolo(txt)+json三种格式标签.zip
为了实现老师、小红和小明这三个并发进程的同步,我们可以使用互斥锁(mutex)、条件变量(condition_variable)以及信号量(semaphore)。这里是一个简化的C++实现:
```cpp
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
#include <vector>
enum Color { RED, GREEN };
class Box {
public:
void put_ball(Color color) {
std::unique_lock<std::mutex> lock(mutex);
balls.push_back(color);
if (balls.empty()) {
signal.put(1); // 放置一个球,唤醒等待的小红或小明
}
lock.unlock();
}
void take_ball(Color color_needed) {
std::unique_lock<std::mutex> lock(mutex);
while (balls.empty() || balls.back() != color_needed) { // 等待正确的颜色
wait_color.wait(lock, [this] { return !balls.empty() && balls.back() == color_needed; });
}
balls.pop_back(); // 取球
lock.unlock();
}
private:
std::queue<Color> balls;
std::mutex mutex;
std::condition_variable wait_color;
std::semaphore signal{1}; // 用于控制放球和取球的顺序
};
int main() {
Box box;
std::thread teacher_thread([=] {
for (int i = 0; i < 5; ++i) {
std::this_thread::sleep_for(std::chrono::seconds(1)); // 模拟老师放置球
box.put_ball((i % 2 == 0) ? Color::RED : Color::GREEN);
}
});
std::thread student_threads[2];
student_threads[0] = std::thread([=] {
box.take_ball(Color::RED);
}, std::ref(box));
student_threads[1] = std::thread([=] {
box.take_ball(Color::GREEN);
}, std::ref(box));
teacher_thread.join();
for (auto& t : student_threads) {
t.join();
}
return 0;
}
```
在这个例子中,我们使用了信号量`signal`来控制放球和取球的顺序,互斥锁`mutex`保护共享数据,条件变量`wait_color`让线程在正确颜色的球出现时进入等待状态。
阅读全文
相关推荐
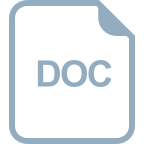
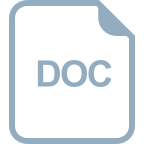















