Unity3D 的摄像机
时间: 2024-06-06 08:05:44 浏览: 21
Unity3D 的摄像机是游戏中用于观察场景的一个组件。它可以设置不同的视角、位置、大小和方向等属性,来实现不同的游戏效果,如第一人称视角、第三人称视角、平面视角等。同时,它还可以通过脚本控制来实现动态的跟随、旋转、缩放等操作。在游戏制作中,摄像机通常被放置在一个空对象下,以便更方便地进行控制和移动。
相关问题
unity3d摄像机输出图片代码
以下是 Unity3D 摄像机输出图片的代码示例:
```csharp
using UnityEngine;
public class CameraCapture : MonoBehaviour
{
public Camera cameraToCapture;
public string savePath = "Assets/CapturedImage.png";
void Update()
{
if (Input.GetKeyDown(KeyCode.Space))
{
Capture();
}
}
void Capture()
{
RenderTexture renderTexture = cameraToCapture.targetTexture;
Texture2D texture = new Texture2D(renderTexture.width, renderTexture.height, TextureFormat.RGB24, false);
cameraToCapture.Render();
RenderTexture.active = renderTexture;
texture.ReadPixels(new Rect(0, 0, renderTexture.width, renderTexture.height), 0, 0);
texture.Apply();
RenderTexture.active = null;
byte[] bytes = texture.EncodeToPNG();
System.IO.File.WriteAllBytes(savePath, bytes);
Debug.Log("Image captured and saved to " + savePath);
}
}
```
这段代码可以让你在 Unity3D 中捕获摄像机的输出图像,并将其保存为 PNG 图片。你可以将其添加到一个空对象上,并将需要捕获的摄像机指定为 `cameraToCapture` 变量。按下空格键即可触发捕获操作。
unity3d摄像机跟随人物
在Unity中实现摄像机跟随人物,可以使用以下两种方式:
1. 通过脚本控制摄像机位置和旋转
可以在摄像机上添加一个脚本,通过脚本代码实现摄像机跟随人物的效果。具体实现步骤如下:
1)获取人物的Transform组件,即人物的位置和旋转信息。
2)将摄像机的位置和旋转信息设置为人物的位置和旋转信息,可以通过Vector3.Lerp()方法实现平滑跟随。
3)在Update()方法中不断更新摄像机的位置和旋转信息,从而实现摄像机的跟随效果。
示例代码:
```
public class CameraFollow : MonoBehaviour {
public Transform target; // 人物的Transform组件
public float smoothTime = 0.3f; // 平滑跟随时间
private Vector3 velocity = Vector3.zero;
void Update() {
// 计算摄像机新的位置
Vector3 targetPosition = target.TransformPoint(new Vector3(0, 5, -10));
// 平滑跟随
transform.position = Vector3.SmoothDamp(transform.position, targetPosition, ref velocity, smoothTime);
// 计算摄像机新的旋转角度
transform.LookAt(target);
}
}
```
2. 使用Unity的跟随组件
Unity提供了一个跟随组件(Follow),可以通过简单的配置实现摄像机跟随人物的效果。具体实现步骤如下:
1)在摄像机上添加一个跟随组件(Component -> New Script -> Follow)。
2)将跟随组件的Target设置为人物的Transform组件。
3)调整跟随组件的其他参数,例如跟随速度、距离等。
示例代码:
```
using UnityEngine;
using UnityStandardAssets.Utility;
public class CameraFollow : MonoBehaviour {
public Transform target; // 人物的Transform组件
public float distance = 10f; // 摄像机与人物的距离
public float height = 5f; // 摄像机与人物的高度
public float damping = 1f; // 跟随速度
private FollowTarget followTarget;
void Start() {
followTarget = GetComponent<FollowTarget>();
followTarget.target = target;
followTarget.distance = distance;
followTarget.height = height;
followTarget.damping = damping;
}
}
```
相关推荐
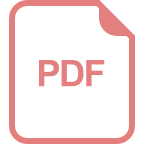
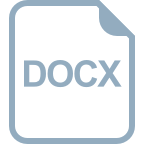












