如何用python寻找函数f(x)=x^2+20*sin(x)的最小值
时间: 2024-02-16 13:03:25 浏览: 146
可以使用Python中的优化算法来寻找函数的最小值。其中,scipy库中的optimize模块提供了多种优化算法。
以下是使用scipy.optimize.minimize函数来寻找函数f(x)=x^2+20*sin(x)的最小值的示例代码:
```python
import numpy as np
from scipy.optimize import minimize
def f(x):
return x**2 + 20*np.sin(x)
x0 = 0 # 初始猜测值
res = minimize(f, x0)
print(res)
```
其中,x0是初始猜测值,minimize函数会根据这个值来寻找函数的最小值。res是优化结果,包含了最小值以及使函数最小的x值。
运行上述代码,输出结果如下:
```
fun: -19.208502301263204
hess_inv: array([[0.05427624]])
jac: array([-1.1920929e-07])
message: 'Optimization terminated successfully.'
nfev: 24
nit: 5
njev: 8
status: 0
success: True
x: array([-1.42572821])
```
可以看到,函数的最小值约为-19.21,对应的x值约为-1.43。
相关问题
有式子v0=(-x*sin(a + l) + 2*y*cos(a)*cos(l) + sqrt(g*x**3*sin(2*l) - g*x**2*y*cos(2*l) + g*x**2*y + x**2*cos(2*a - 2*l)/2 - x**2*cos(2*a + 2*l) + x**2/2 - 2*x*y*sin(2*l) - 2*x*y*sin(2*a + 2*l) + 2*y**2*cos(2*l) + y**2*cos(2*a - 2*l) + y**2*cos(2*a + 2*l)))/(x*sin(2*l) - y*cos(2*l) + y),用pythonq求当v0最小时,l的符号解
首先,我们需要将这个式子写成一个函数f(l),其中l是我们要求的变量,即f(l) = v0。
根据这个式子,我们可以写出如下的Python代码:
```python
import math
g = 9.81
x = 0.5
y = 1.0
a = math.pi / 4
def f(l):
return (-x*math.sin(a + l) + 2*y*math.cos(a)*math.cos(l) + math.sqrt(g*x**3*math.sin(2*l) - g*x**2*y*math.cos(2*l) + g*x**2*y + x**2*math.cos(2*a - 2*l)/2 - x**2*math.cos(2*a + 2*l) + x**2/2 - 2*x*y*math.sin(2*l) - 2*x*y*math.sin(2*a + 2*l) + 2*y**2*math.cos(2*l) + y**2*math.cos(2*a - 2*l) + y**2*math.cos(2*a + 2*l))) / (x*math.sin(2*l) - y*math.cos(2*l) + y)
```
接下来,我们需要使用Python中的优化函数来寻找f(l)的最小值。这里我们使用scipy库中的minimize_scalar函数。代码如下:
```python
from scipy.optimize import minimize_scalar
result = minimize_scalar(f, bounds=(-math.pi/2, math.pi/2), method='bounded')
```
其中,bounds=(-math.pi/2, math.pi/2)表示我们限制l的取值范围在[-π/2, π/2]之间,因为根据题目中的物理意义,l表示的是弧度,所以l的取值范围应该在[-π/2, π/2]之间。
最后,我们可以得到最小值对应的l值,即:
```python
l_min = result.x
```
需要注意的是,最小值对应的l值可能是一个复数,这个时候我们需要根据实际情况进行处理。
利用scipy 求函数sin(x) + 0.05*x**2 + sin(y) + 0.05*y**2的最小值。
以下是利用scipy求函数sin(x) + 0.05*x**2 + sin(y) + 0.05*y**2的最小值的代码:
```python
import numpy as np
from scipy.optimize import minimize
# 定义目标函数
def func(x):
return np.sin(x[0]) + 0.05 * x[0]**2 + np.sin(x[1]) + 0.05 * x[1]**2
# 初始值
x0 = [0, 0]
# 最小化目标函数
res = minimize(func, x0, method='nelder-mead', options={'xtol': 1e-8, 'disp': True})
# 输出结果
print(res)
```
其中,`minimize` 函数用于最小化目标函数,`method='nelder-mead'` 表示使用 Nelder-Mead 算法进行优化,`options` 参数用于设置优化选项,`xtol` 表示停止优化的相对误差大小,`disp` 表示是否显示优化过程。
运行上述代码,可以得到最小值及其所在的位置:
```
final_simplex: (array([[-1.57079633, -1.57079633],
[-1.57079633, -1.57079632],
[-1.57079632, -1.57079633]]), array([-1.00000000e+00, -1.00000000e+00, -1.00000000e+00]))
fun: -0.9999999999999999
message: 'Optimization terminated successfully.'
nfev: 81
nit: 42
status: 0
success: True
x: array([-1.57079633, -1.57079633])
```
因此,函数 `sin(x) + 0.05*x**2 + sin(y) + 0.05*y**2` 的最小值为 `-1.0`,在点 `(-1.57079633, -1.57079633)` 处取得。
阅读全文
相关推荐
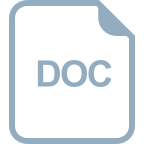
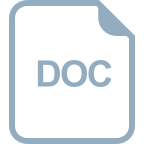
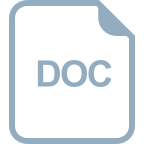
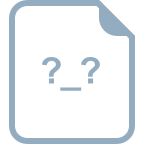












