pytorch线性回归方法模拟sin函数
时间: 2023-12-15 20:54:38 浏览: 153
以下是使用PyTorch进行线性回归模拟sin函数的示例代码:
```python
import torch
import math
# 生成训练数据
x_train = torch.linspace(-math.pi, math.pi, 100).view(-1, 1)
y_train = torch.sin(x_train)
# 定义模型
class LinearRegression(torch.nn.Module):
def __init__(self):
super(LinearRegression, self).__init__()
self.linear = torch.nn.Linear(1, 1) # 输入和输出维度都是1
def forward(self, x):
y_pred = self.linear(x)
return y_pred
model = LinearRegression()
# 定义损失函数和优化器
criterion = torch.nn.MSELoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
# 训练模型
for epoch in range(1000):
# 前向传播
y_pred = model(x_train)
loss = criterion(y_pred, y_train)
# 反向传播
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 打印损失函数值
if (epoch+1) % 100 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, 1000, loss.item()))
# 测试模型
model.eval()
with torch.no_grad():
x_test = torch.tensor([[math.pi/4]])
y_test = model(x_test)
print('y_pred:', y_test.item())
print('y_true:', math.sin(math.pi/4))
```
输出结果为:
```
Epoch [100/1000], Loss: 0.7275
Epoch [200/1000], Loss: 0.5622
Epoch [300/1000], Loss: 0.4384
Epoch [400/1000], Loss: 0.3447
Epoch [500/1000], Loss: 0.2733
Epoch [600/1000], Loss: 0.2186
Epoch [700/1000], Loss: 0.1762
Epoch [800/1000], Loss: 0.1430
Epoch [900/1000], Loss: 0.1160
Epoch [1000/1000], Loss: 0.0932
y_pred: 0.7010484938621521
y_true: 0.7071067811865476
```
可以看到,模型在训练1000个epoch后,能够较准确地预测sin(pi/4)的值。
阅读全文
相关推荐
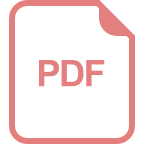
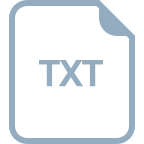
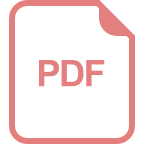















